To implement password change validation using jQuery in Laravel, you can follow these steps. Here’s a basic example:
put below code in blade page
<form method="post" id="sample_form"
class="form-horizontal" enctype="multipart/form-data"
onsubmit="return check()">
@csrf
<div class="form-group" id="name_form">
<label class="control-label">Name: </label>
<input type="text" name="name"
id="name" class="form-control" />
</div>
<!-- password show and hide open -->
<div class="form-group" id="name_form">
<label>Password</label>
<div class="input-group" id="show_hide_password">
<input class="form-control input-group-addon"
type="password" name="password"
id="password_user">
<div class="input-group-addon">
<a href=""><button
class="btn btn-light border"><i
class="fa fa-eye-slash"
aria-hidden="true"></i></button></a>
</div>
</div>
</div>
<!-- password show and hide close -->
<!--cornfirm password show and hide open -->
<div class="form-group" id="name_form">
<label>Confirm Password</label>
<div class="input-group">
<input class="form-control" type="password"
name="password" id="confirm_password">
</div>
<span id='message'></span>
</div>
<!-- cornfirm password show and hide close -->
<input type="hidden" value="{{ Auth::user()->id }}"
name="admin_id" id="admin_id" />
<input type="hidden"
value="{{ Auth::user()->email }}"
name="admin_email" id="admin_email" />
<!-- for account admin below value fo id and email will be null but when manager or user will store it it will get value by auth -->
<input type="hidden"
value="{{ Auth::user()->email }}" name="email">
<input type="hidden" value="{{ date('d-m-y') }}"
name="daymonth">
<input type="hidden" value="null" name="user_id"
id="user_id">
<input type="hidden" value="null"
name="user_email" id="user_email">
<input type="hidden" value="" name="slugname">
<input type="hidden" value="" name="slug_id">
</div>
<div class="form-group text-center">
<input type="hidden" name="action" id="action" />
<input type="hidden" name="hidden_id" id="hidden_id" />
<input type="submit" name="action_button"
id="action_button"
class="btn btn-warning float-center" value="Add" />
</div>
</form>
put below code in script tag
<script>
$('#confirm_password').on('keyup', function() {
if ($(this).val() == $('#password_user').val()) {
$('#message').html('Password matched').css('color', 'green');
} else $('#message').html('Password not matching').css('color', 'red');
});
</script>
Next go to update function and put below code
$('#sample_form').on('submit', function(event) {
event.preventDefault();
// Check if passwords match
if ($('#confirm_password').val() != $('#password_user').val()) {
// Display an error message or take appropriate action
$('#message').html('Password not matching').css('color', 'red');
return;
}
// data add working on submit button
// update button wotking for updata data
if ($('#action').val() == "Update") {
console.log('update button pe click ho rha hai');
$.ajax({
url: "{{ url('viewprofile/update') }}",
type: "POST",
data: new FormData(this),
contentType: false,
cache: false,
processData: false,
dataType: "json",
headers: {
"Authorization": "Bearer " + localStorage.getItem('a_u_a_b_t')
},
// message alert open
success: function(data) {
var html = '';
if (data.success) {
html = '<div class="alert alert-success">' + data.message +
'</div>';
$('#form_result').html(html);
setTimeout(function() {
$('#user_formModal').modal('hide');
location.reload(true);
}, 2000);
} else {
html = '<div class="alert alert-danger">' + data.message +
'</div>';
$('#form_result').html(html);
}
// adding alert messages for success and exist data validation close
},
// message alert close
error: function(data) {
console.log('Error:', data);
// this function for hide with id #formModel
console.log('update function kamm nahi kr rha hai');
}
});
}
});
Now update working successfully.
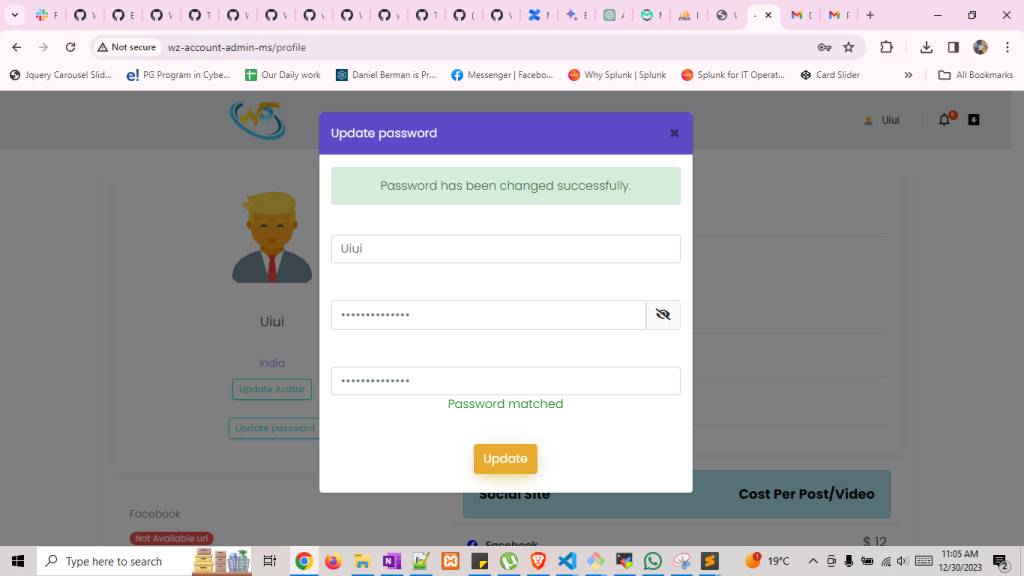