Multi auth in Laravel is a feature that allows you to have multiple authentication guards in your application. This means that you can have different login systems for different types of users, such as Admin vendor and user.
Install Project
composer create-project laravel/laravel multi-auth
After installation go to user table and put below like this.
public function up(): void
{
Schema::create('users', function (Blueprint $table) {
$table->id();
$table->string('name');
$table->string('username')->nullable();
$table->string('email')->unique();
$table->timestamp('email_verified_at')->nullable();
$table->string('password');
$table->string('photo')->nullable();
$table->string('phone')->nullable();
$table->text('address')->nullable();
$table->enum('role',['admin','vendor','user'])->default('user');
$table->enum('status',['active','inactive'])->default('active');
$table->rememberToken();
$table->timestamps();
});
}
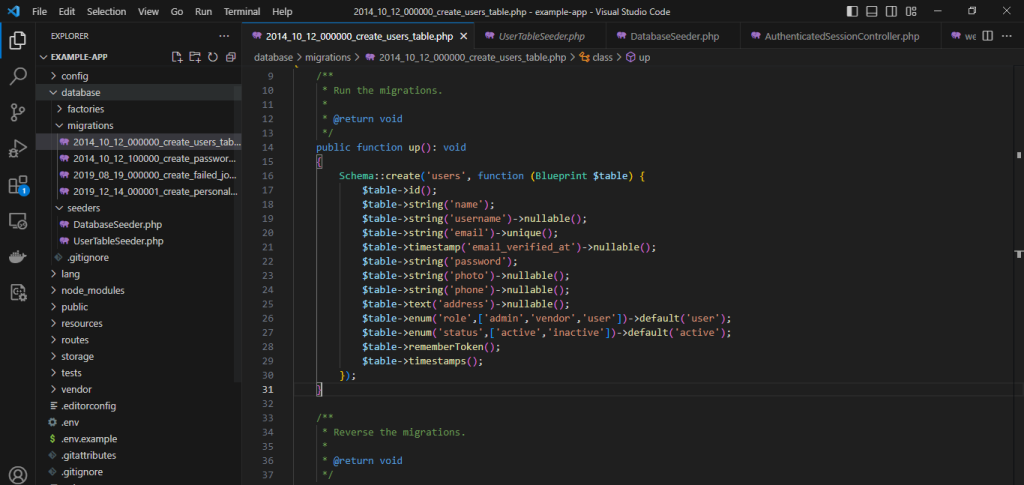
Next go to .env file and put your database name
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=wz_account_admin_ms
DB_USERNAME=root
DB_PASSWORD=
Next to create seeders table
php artisan make:seeder UserTableSeeder
And put below code as well
public function run(): void
{
DB::table('users')->insert([
// admin
[
'name'=> 'Admin',
'username'=> 'admin',
'email'=> 'admin@gmail.com',
'password'=> Hash::make('111'),
'role'=> 'admin',
'status'=> 'active',
],
// vendor
[
'name' => 'Ariyan Vendor',
'username'=> 'vendor',
'email'=> 'vendor@gmail.com',
'password'=> Hash::make(111),
'role'=> 'vendor',
'status'=> 'active',
],
[
'name' => 'User',
'username'=> 'user',
'email'=> 'user@gmail.com',
'password'=> Hash::make(111),
'role'=> 'user',
'status'=> 'active',
],
]);
}
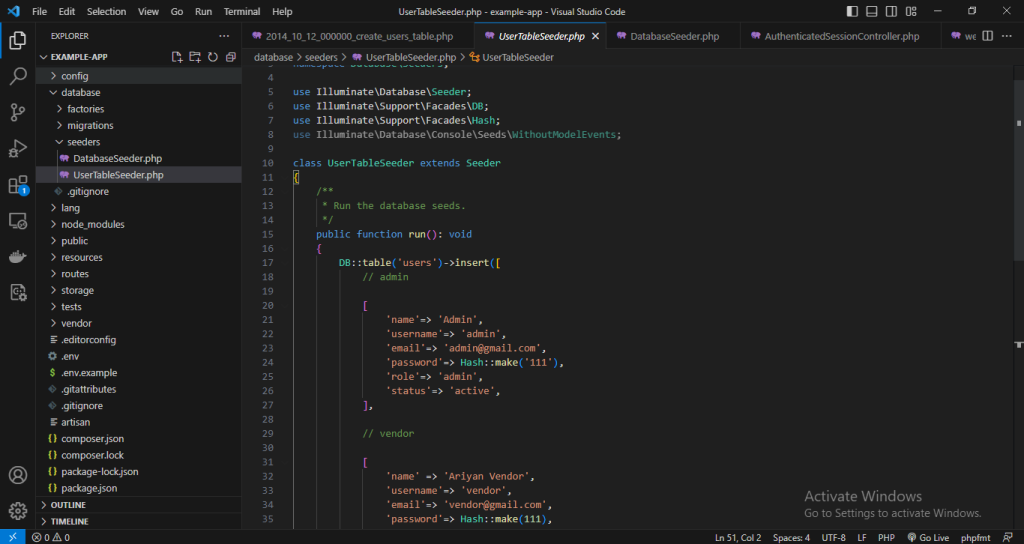
Next go to DatabaseSeeder and put below code
$this->call(UserTableSeeder::class);
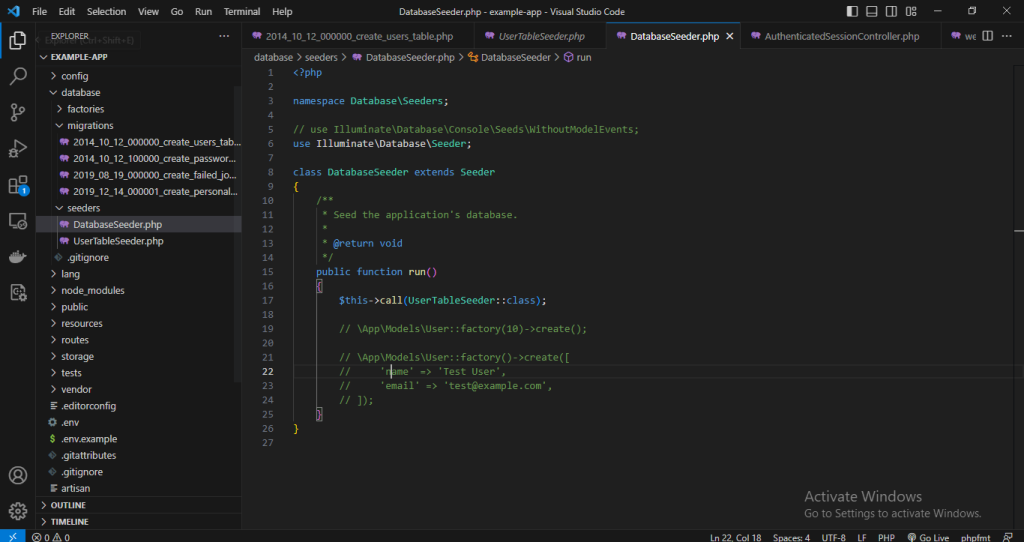
Next to run below command
php artisan migrate
php artisan db:seed
Next to create controller
Php artisan make:controller AdminController
Php artisan make:controller VendorController
Got to AdminController and put below code
public function AdminDashboard(){
return view('admin.admin_dashboard');
}
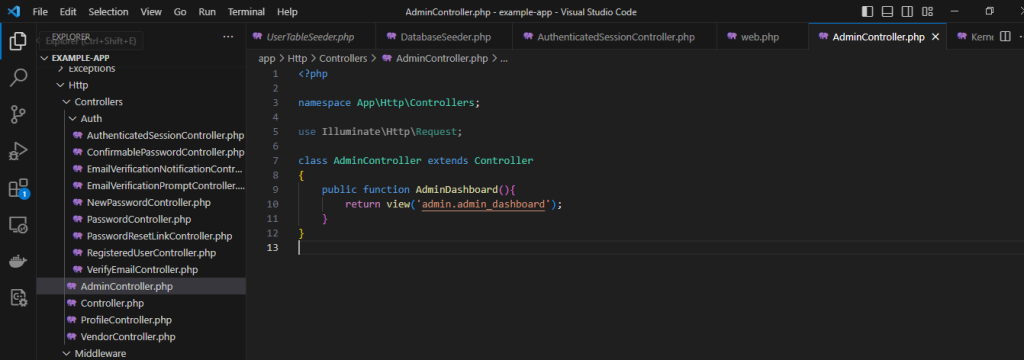
Next go to vendorController and put below code
public function VendorDashboard(){
return view('vendor.vendor_dashboard');
}
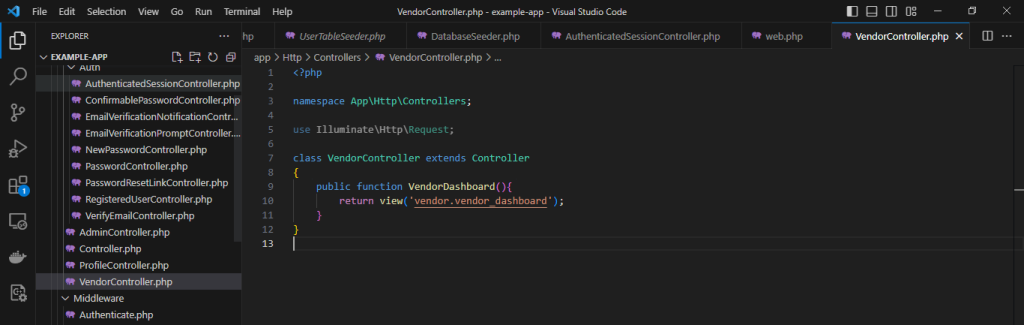
And check all migration would be gone in database
Next to create middleware
php artisan make:middleware Role
Go to your generated middleware and put below code
public function handle(Request $request, Closure $next, $role)
{
if($request->user()->role !== $role){
return redirect('dashboard');
}
return $next($request);
}
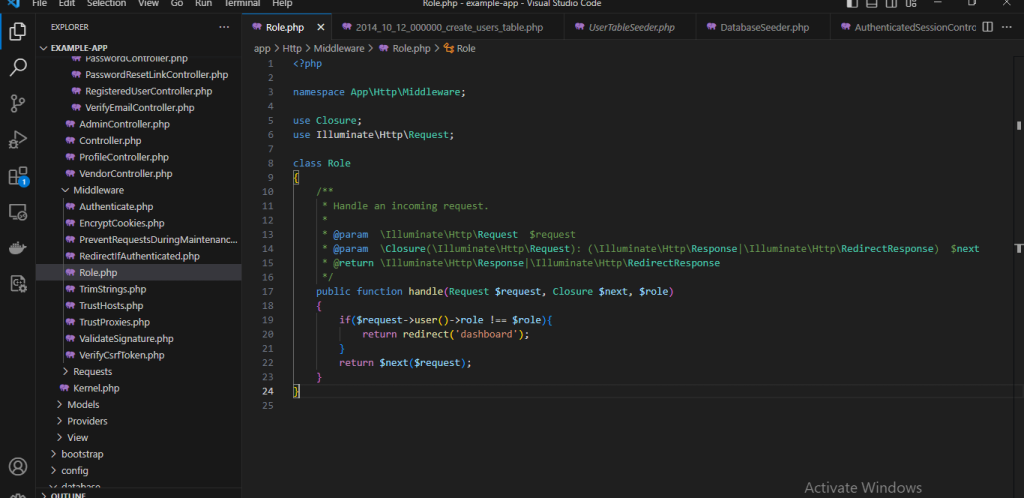
Next go to kernal.php and put below code in routemiddleware
'role' => \App\Http\Middleware\Role::class,
Next to web.php and put below code
Route::middleware(['auth', 'role:admin'])->group(function() {
Route::get('/admin/dashboard', [AdminController::class, 'AdminDashboard'])->name('admin.dashboard');
});
Route::middleware(['auth', 'role:vendor'])->group(function() {
Route::get('/vendor/dashboard', [VendorController::class, 'VendorDashboard'])->name('vendor.dashboard');
});
Next to go AuthenticatedSessionController and update below code
public function store(LoginRequest $request): RedirectResponse
{
$request->authenticate();
$request->session()->regenerate();
$url = '';
if($request->user()->role === 'admin'){
Log::info("admin ke if me in kr gye hai");
$url = '/admin/dashboard';
// return redirect()->route('admin.dashboard');
} elseif($request->user()->role === 'vendor'){
Log::info("vendor ke if me in kr gye hai");
$url = 'vendor/dashboard';
} elseif($request->user()->role === 'user'){
Log::info("user ke if me in kr gye hai");
$url = '/dashboard';
}
return redirect()->intended($url);
}
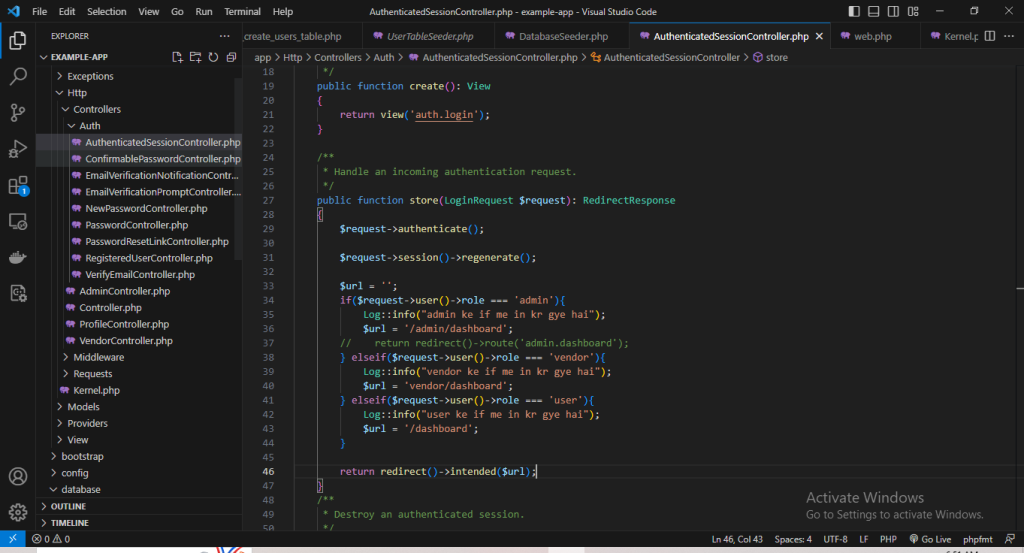
Now php artisan serve
All setup successfully
Admin Login
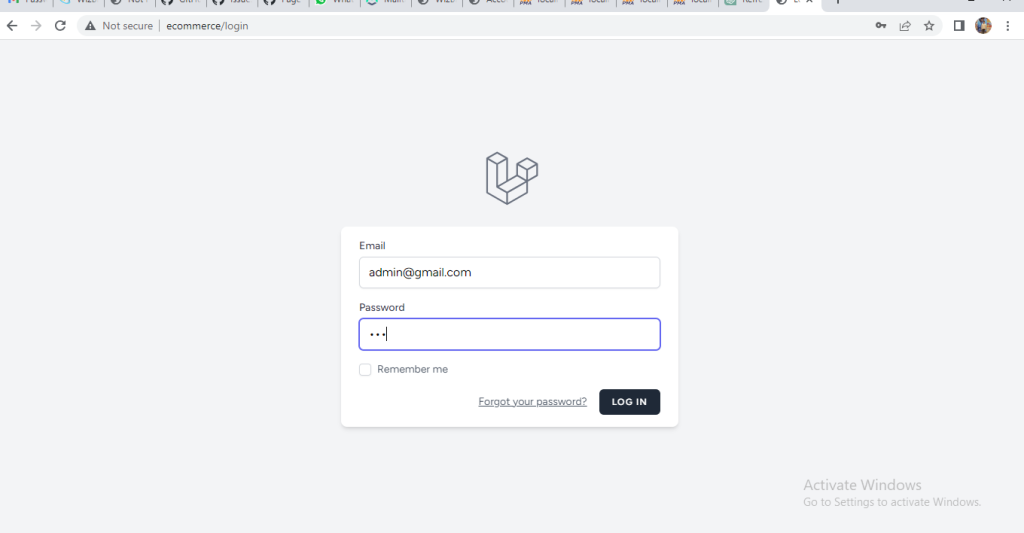
Now dashboard coming redirect successfully
Thanks its working now 👍
[…] Multi Auth Login in laravel Admin Vendor and User? […]