In this tutorial we’re going to learn how to store JSON data in Database in Laravel
What is JSON ?
JSON (JavaScript Object Notation) is a lightweight, text-based format for data exchange that is easy for humans to read and write and easy for machines to parse and generate. JSON is a popular data format used in web applications, APIs, and data exchange between systems. JSON data is represented as key-value pairs and uses a syntax similar to JavaScript object literals. Each key-value pair is separated by a comma and enclosed in curly braces
Step 1: Install Laravel
Use Below command for installation
composer create-project laravel/laravel example-app
Step 2: Create Migration
php artisan make:migration create_items_table
Next go to migration file and put below code
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
return new class extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up(): void
{
Schema::create('items', function (Blueprint $table) {
$table->id();
$table->string('title');
$table->json('data')->nullable();
$table->timestamps();
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down(): void
{
Schema::dropIfExists('items');
}
};
Then run migration command to create items table.
php artisan migrate
Next to create migration file
php artisan make:model Item
Next go to model and put below code
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Database\Eloquent\Model;
use Illuminate\Database\Eloquent\Casts\Attribute;
class Item extends Model
{
use HasFactory;
/**
* Write code on Method
*
* @return response()
*/
protected $fillable = [
'title', 'data'
];
/**
* Get the user's first name.
*
* @return \Illuminate\Database\Eloquent\Casts\Attribute
*/
protected function data(): Attribute
{
return Attribute::make(
get: fn ($value) => json_decode($value, true),
set: fn ($value) => json_encode($value),
);
}
}
Next got to web.php and put below code
<?php
use Illuminate\Support\Facades\Route;
use App\Http\Controllers\ItemController;
/*
|--------------------------------------------------------------------------
| Web Routes
|--------------------------------------------------------------------------
|
| Here is where you can register web routes for your application. These
| routes are loaded by the RouteServiceProvider within a group which
| contains the "web" middleware group. Now create something great!
|
*/
Route::get('item', [ItemController::class, 'index']);
Next go to Controller file and put below code
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\Models\Item;
class ItemController extends Controller
{
/**
* Write code on Method
*
* @return response()
*/
public function index()
{
$input = [
'title' => 'Demo Title',
'data' => [
'1' => 'One',
'2' => 'Two',
'3' => 'Three'
]
];
$item = Item::create($input);
dd($item->data);
}
}
Now run the project
php artisan serve
Open this url
http://127.0.0.1:8000/item
Output:-
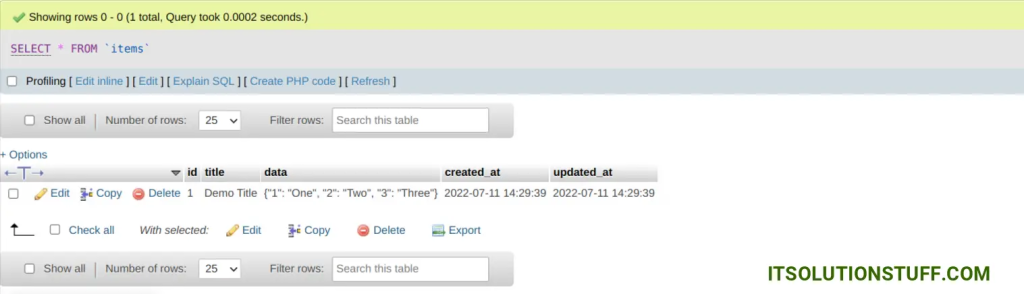
Now data store successfully.
[…] Laravel 10 Store JSON in Database Example ? […]