A JavaScript constructor function is a function that is used to create new objects. It is called a constructor because it constructs new objects.
To create a JavaScript constructor function, you use the function
keyword. The function name should be descriptive of the type of object that the function will create. For example, a constructor function for a Person
object might be called Person
.
The body of the constructor function should contain the code that is executed when a new object is created. This code can be used to initialize the properties of the new object.
Here is an example of a JavaScript constructor function that creates a Person
object:
<script type="text/javascript">
function Person(person_name, person_age, person_gender) {
// assigning parameter values to the calling object
this.name = person_name,
this.age = person_age,
this.gender = person_gender,
this.greet = function() {
return ('Hi' + ' ' + this.name);
}
}
// creating objects
const person1 = new Person('Amit', 23, 'male');
const person2 = new Person('Sumit', 25, 'female');
// accessing properties
console.log(person1.name);
console.log(person2.name);
</script>
Output:-
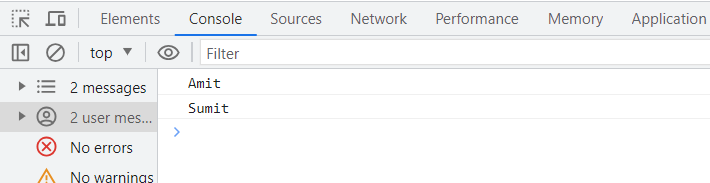