In this tutorial I’m going to solve how to use Toaster notification in laravel when condition is execute.
First use below cdn
<link rel="stylesheet" type="text/css" href="https://cdnjs.cloudflare.com/ajax/libs/toastr.js/latest/toastr.min.css">
<script src="https://cdnjs.cloudflare.com/ajax/libs/toastr.js/latest/js/toastr.min.js"></script>
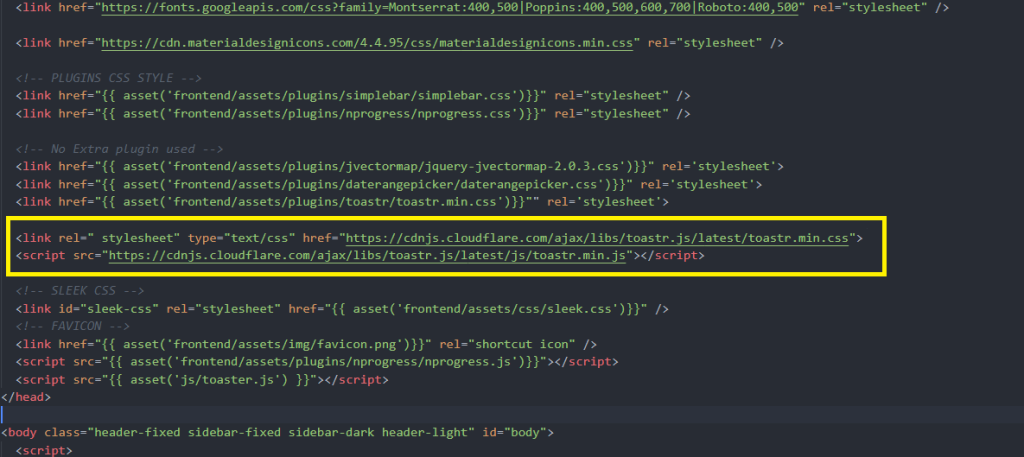
Then put below code in script tag like below.
<script>
@if(Session::has('message'))
toastr.options =
{
"closeButton" : true,
"progressBar" : true
}
toastr.success("{{ session('message') }}");
@endif
@if(Session::has('error'))
toastr.options =
{
"closeButton" : true,
"progressBar" : true
}
toastr.error("{{ session('error') }}");
@endif
@if(Session::has('info'))
toastr.options =
{
"closeButton" : true,
"progressBar" : true
}
toastr.info("{{ session('info') }}");
@endif
@if(Session::has('warning'))
toastr.options =
{
"closeButton" : true,
"progressBar" : true
}
toastr.warning("{{ session('warning') }}");
@endif
</script>
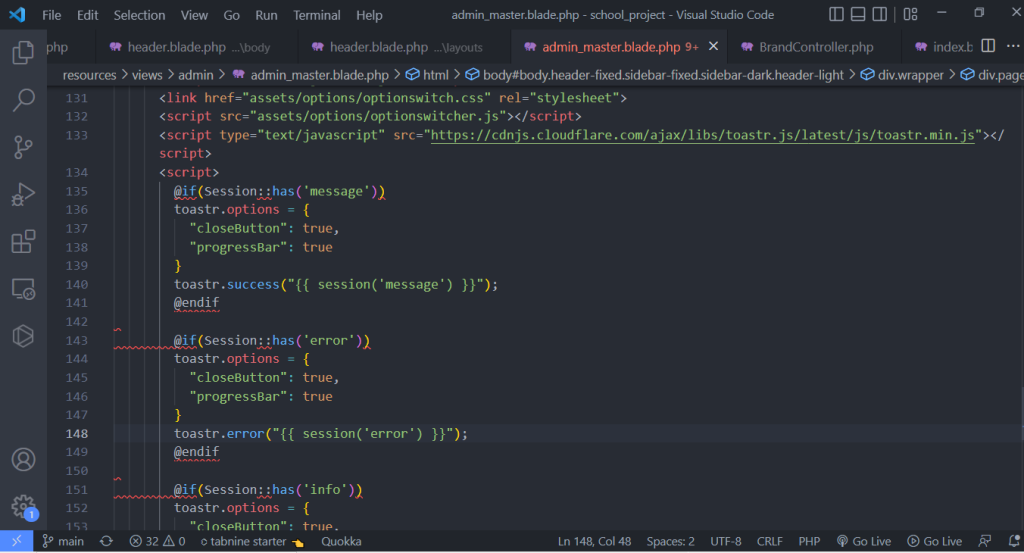
Next go to blade page and put below code
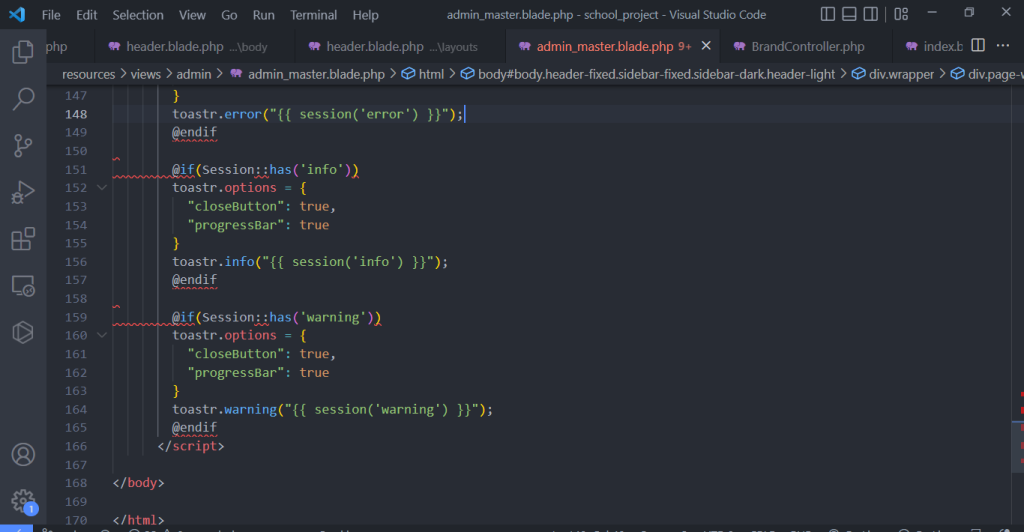
Next go to controller and put below code.
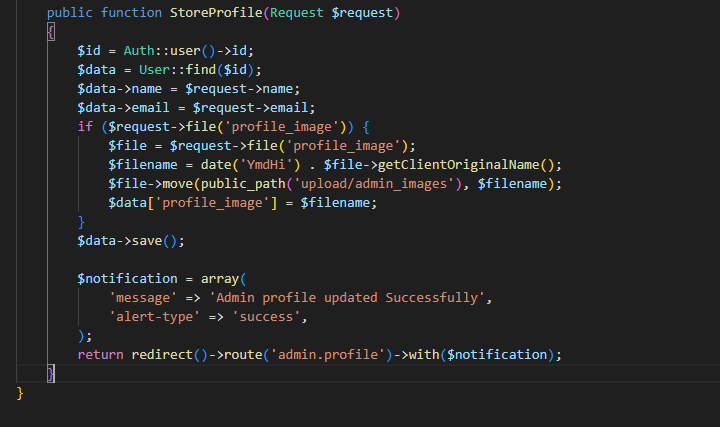
$notification = array(
'message' => 'Brand Inserted Successfully',
'alert-type' => 'success',
);
return redirect()->back()->with($notification);
Now toastr set successfully.
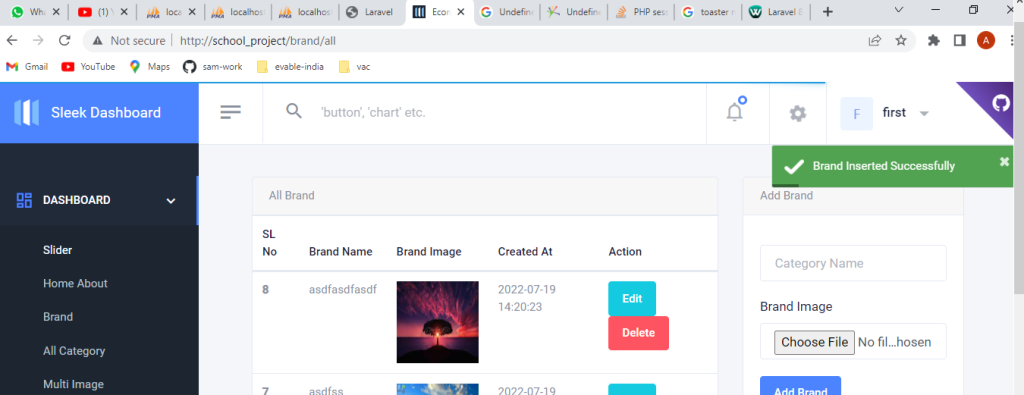