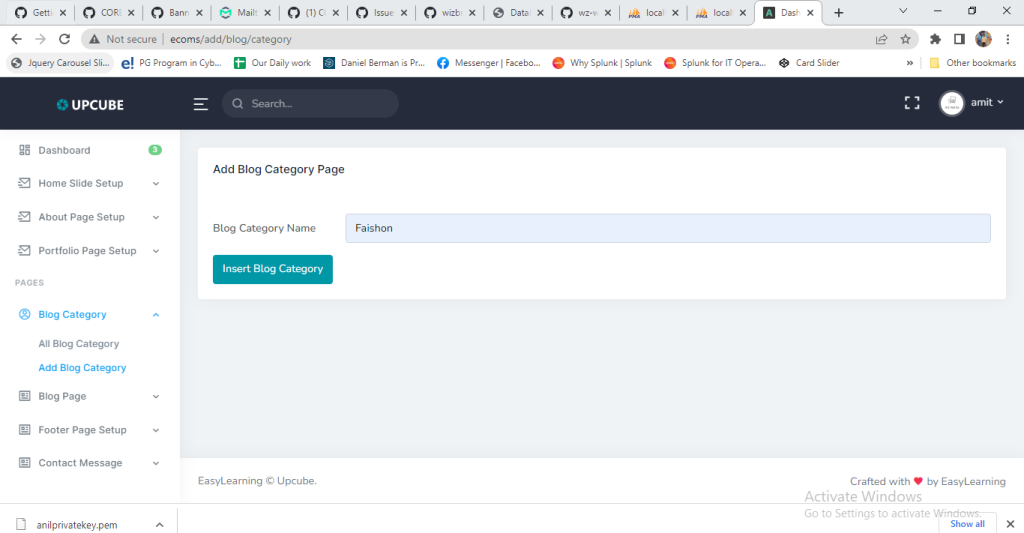
In this tutorial I’m going to insert category system in laravel in very easy way follow this video we ‘have demonstrated in very easy way.
Php artisan make:model BlogCategory -m
Next go to migration file and paste below code.
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Database\Eloquent\Model;
class BlogCategory extends Model
{
use HasFactory;
protected $guarded = [];
}
Next go to migration file and paste below code.
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
return new class extends Migration
{
/**
* Run the migrations.
*/
public function up(): void
{
Schema::create('blog_categories', function (Blueprint $table) {
$table->id();
$table->string('blog_category')->nullable();
$table->timestamps();
});
}
/**
* Reverse the migrations.
*/
public function down(): void
{
Schema::dropIfExists('blog_categories');
}
};
Next create controller and paste below code
php artisan make:controller Home/BlogCategoryController
<?php
namespace App\Http\Controllers\Home;
use App\Http\Controllers\Controller;
use App\Models\BlogCategory;
use Illuminate\Http\Request;
class BlogCategoryController extends Controller
{
public function AllBlogCategory(){
$blogcategory = BlogCategory::latest()->get();
return view('admin.blog_category.blog_category_all',compact('blogcategory'));
}
public function AddBlogCategory()
{
return view('admin.blog_category.blog_category_add');
}
public function StoreBlogCategory(Request $request)
{
$request->validate([
'blog_category' => 'required',
], [
'blog_category.required' => 'Blog Cateogry Name is Required',
]);
BlogCategory::insert([
'blog_category' => $request->blog_category,
]);
$notification = array(
'message' => 'Blog Category Inserted Successfully',
'alert-type' => 'success',
);
return redirect()->route('all.blog.category')->with($notification);
}
public function EditBlogCategory($id)
{
$blogcategory = BlogCategory::findOrFail($id);
return view('admin.blog_category.blog_category_edit', compact('blogcategory'));
}
public function UpdateBlogCategory(Request $request, $id)
{
BlogCategory::findOrFail($id)->update([
'blog_category' => $request->blog_category,
]);
$notification = array(
'message' => 'Blog Category Updated Successfully',
'alert-type' => 'success',
);
return redirect()->route('all.blog.category')->with($notification);
} // End Method
public function DeleteBlogCategory($id)
{
BlogCategory::findOrFail($id)->delete();
$notification = array(
'message' => 'Blog Category Deleted Successfully',
'alert-type' => 'success',
);
return redirect()->back()->with($notification);
}
}
Next go to view page and paste below code
@extends('admin.admin_master')
@section('admin')
<div class="page-content">
<div class="container-fluid">
<!-- start page title -->
<div class="row">
<div class="col-12">
<div class="page-title-box d-sm-flex align-items-center justify-content-between">
<h4 class="mb-sm-0">Blog Category All</h4>
</div>
</div>
</div>
<!-- end page title -->
<div class="row">
<div class="col-12">
<div class="card">
<div class="card-body">
<h4 class="card-title">Blog Category All Data </h4>
<table id="datatable" class="table table-bordered dt-responsive nowrap"
style="border-collapse: collapse; border-spacing: 0; width: 100%;">
<thead>
<tr>
<th>Sl</th>
<th>Blog Category Name</th>
<th>Action</th>
</thead>
<tbody>
@foreach ($blogcategory as $key => $item)
<tr>
<td> {{ $key + 1 }} </td>
<td> {{ $item->blog_category }} </td>
<td>
<a href="{{ route('edit.blog.category', $item->id) }}"
class="btn btn-info sm" title="Edit Data"> <i class="fas fa-edit"></i>
</a>
<a href="{{ route('delete.blog.category', $item->id) }}"
class="btn btn-danger sm" title="Delete Data" id="delete"> <i
class="fas fa-trash-alt"></i> </a>
</td>
</tr>
@endforeach
</tbody>
</table>
</div>
</div>
</div> <!-- end col -->
</div> <!-- end row -->
</div> <!-- container-fluid -->
</div>
@endsection
Next go to route and paste below code
Route::controller(BlogCategoryController::class)->group(function () {
Route::get('/all/blog/category', 'AllBlogCategory')->name('all.blog.category');
Route::get('/add/blog/category', 'AddBlogCategory')->name('add.blog.category');
Route::post('/store/blog/category', 'StoreBlogCategory')->name('store.blog.category');
Route::get('/edit/blog/category/{id}', 'EditBlogCategory')->name('edit.blog.category');
Route::post('/update/blog/category/{id}', 'UpdateBlogCategory')->name('update.blog.category');
Route::get('/delete/blog/category/{id}', 'DeleteBlogCategory')->name('delete.blog.category');
});
Next add code in your sidebar
<ul class="sub-menu" aria-expanded="false">
<li><a href="{{ route('all.blog.category')}}">All Blog Category</a></li>
<li><a href="{{ route('add.blog.category') }}">Add Blog Category</a></li>
</ul>
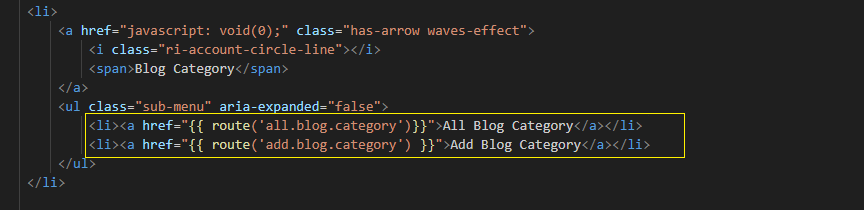
Now all setup is done
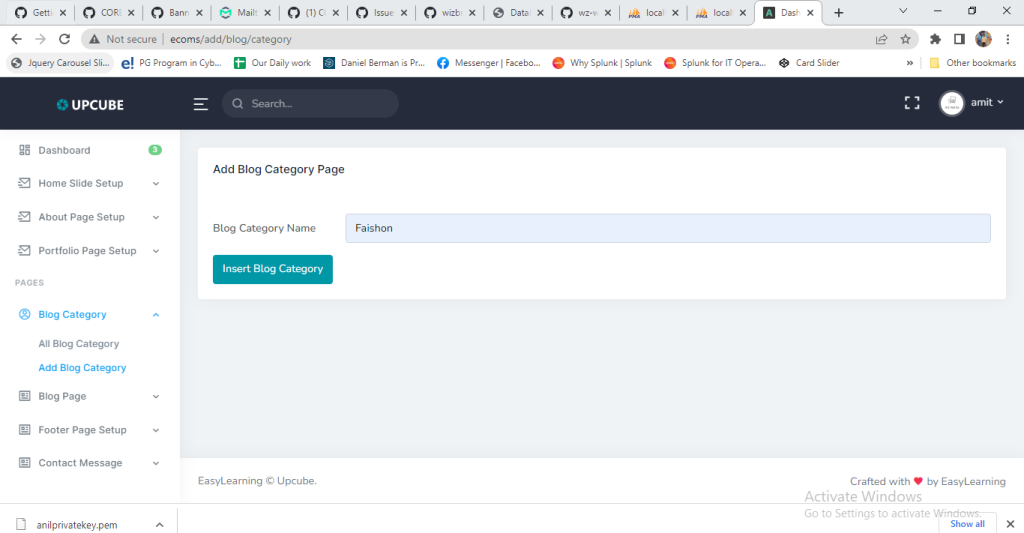
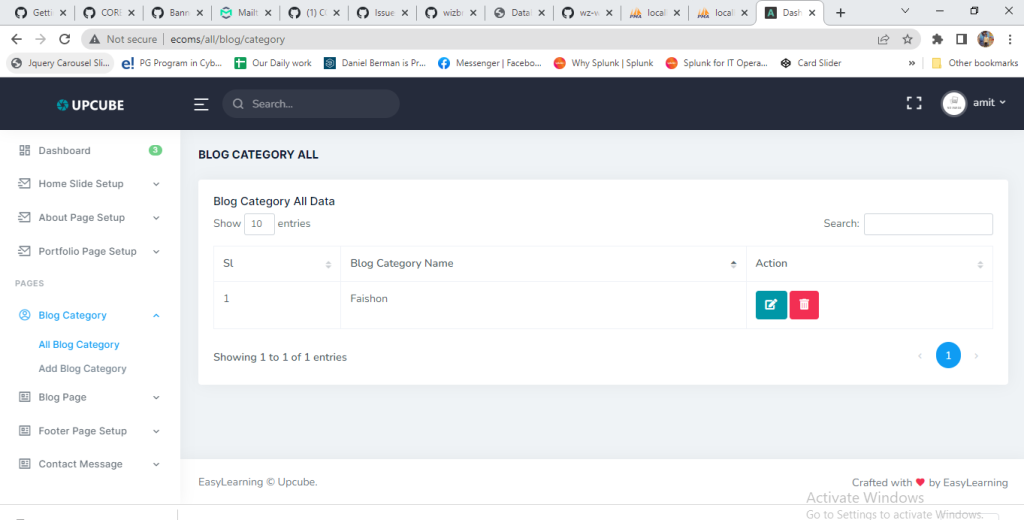
[…] How to Insert Category function in Laravel ? […]