In this tutorial i’m going to learn how to do validation in laravel using controller function. This method automatically validates incoming HTTP requests, and if the validation fails, it will automatically redirect the user back to the form with error messages.
Just go to controller function and put below code
public function StoreBrand(Request $request){
$request->validate([
'brand_name' => 'required',
'brand_image' => 'required|image|mimes:jpeg,png,jpg,gif,svg|max:2048',
]);
$image = $request->file('brand_image');
$name_gen = hexdec(uniqid()).'.'.$image->getClientOriginalExtension();
Image::make($image)->resize(300,300)->save('upload/brand/'.$name_gen);
$save_url = 'upload/brand/'.$name_gen;
Brand::insert([
'brand_name' => $request->brand_name,
'brand_slug' => strtolower(str_replace(' ', '-',$request->brand_name)),
'brand_image' => $save_url,
]);
$notification = array(
'message' => 'Brand Inserted Successfully',
'alert-type' => 'success'
);
return redirect()->route('all.brand')->with($notification);
}
Next go to blade page and put below code.
<form id="myForm" method="post" action="{{ route('store.brand') }}"
enctype="multipart/form-data">
@csrf
<div class="row mb-3">
<div class="col-sm-3">
<h6 class="mb-0">Brand Name</h6>
</div>
<div class="form-group col-sm-9 text-secondary">
<input type="text" name="brand_name" class="form-control" />
@error('brand_name')
<span class="text-danger">{{ $message }}</span>
@enderror
</div>
</div>
<div class="row mb-3">
<div class="col-sm-3">
<h6 class="mb-0">Brand Image </h6>
</div>
<div class="form-group col-sm-9 text-secondary">
<input type="file" name="brand_image" class="form-control" id="image" />
@error('brand_image')
<span class="text-danger">{{ $message }}</span>
@enderror
</div>
</div>
<div class="row mb-3">
<div class="col-sm-3">
<h6 class="mb-0"> </h6>
</div>
<div class="col-sm-9 text-secondary">
<img id="showImage" src="{{ url('upload/no_image.jpg') }}" alt="Admin"
style="width:100px; height: 100px;">
</div>
</div>
<div class="row">
<div class="col-sm-3"></div>
<div class="col-sm-9 text-secondary">
<input type="submit" class="btn btn-primary px-4" value="Save Changes" />
</div>
</div>
</form>
Output:-
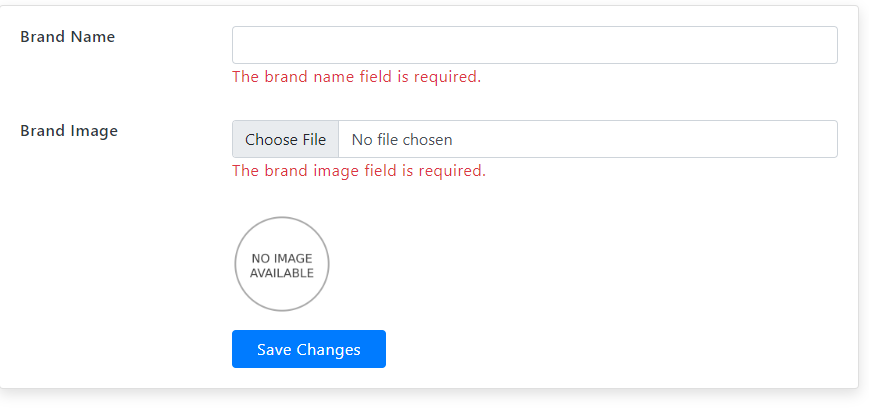