In this tutorial I’m going to use how to delete multiple data using checkbox in laravel. So follow this tutorial I ‘have mentioned in very easy way.
First to install laravel project
composer create-project laravel/laravel laravel-demo
Build Database Connection
To connect laravel with the database i have to put .env file database name.
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=laravel_active
DB_USERNAME=root
DB_PASSWORD=
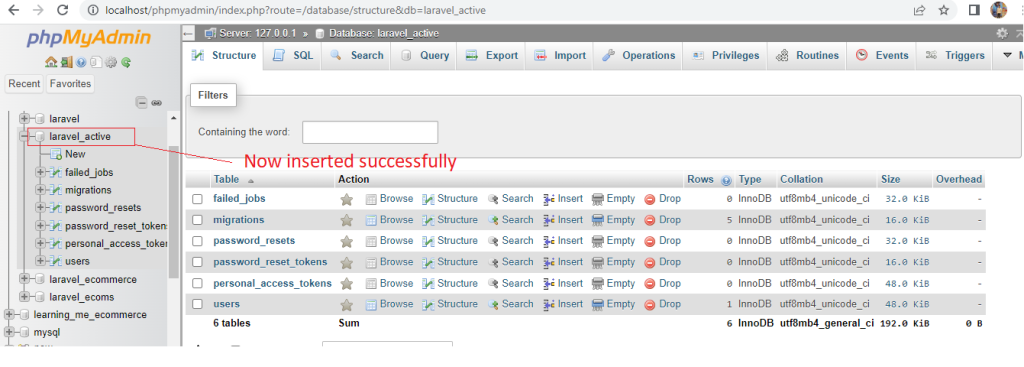
composer requires laravel/ui
php artisan ui bootstrap
php artisan ui bootstrap --auth
Next to run below code.
npm install
npm run dev
Next run the project and store some user data
Next to create the controller file
php artisan make:controller UserController
Next go to controller file and put below code in your controller
<?php
namespace App\Http\Controllers;
use App\Models\User;
use Illuminate\Http\Request;
class UserController extends Controller
{
public function index()
{
$users = User::get();
return view('users',compact('users'));
}
public function removeMulti(Request $request)
{
$ids = $request->ids;
User::whereIn('id',explode(",",$ids))->delete();
return response()->json(['status'=>true,'message'=>"Student successfully removed."]);
}
}
Next got to web.php and put below code
Route::delete('delete-all', [App\Http\Controllers\UserController::class, 'removeMulti']);
Next to build file user.blade.php file
<!DOCTYPE html>
<html lang="{{ str_replace('_', '-', app()->getLocale()) }}">
<head>
<title>Laravel Multi Checkboxes Example</title>
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha1/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-GLhlTQ8iRABdZLl6O3oVMWSktQOp6b7In1Zl3/Jr59b6EGGoI1aFkw7cmDA6j6gD" crossorigin="anonymous">
<meta name="csrf-token" content="{{ csrf_token() }}">
</head>
<body>
<div class="container">
@if ($message = Session::get('success'))
<div class="alert alert-info">
<p>{{ $message }}</p>
</div>
@endif
<h2 class="mb-4">Laravel Checkbox Multiple Rows Delete Example</h2>
<button class="btn btn-primary btn-xs removeAll mb-3">Remove All Data</button>
<table class="table table-bordered">
<tr>
<th><input type="checkbox" id="checkboxesMain"></th>
<th>Name</th>
<th>Email</th>
<th>Status</th>
</tr>
@foreach($users as $user)
<tr id="tr_{{$user->id}}">
<td><input type="checkbox" class="checkbox" data-id="{{$user->id}}"></td>
<td>{{ $user->name }}</td>
<td>{{ $user->email }}</td>
</tr>
@endforeach
</table>
</div>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.2.1/jquery.min.js"></script>
</body>
<script type = "text/javascript" >
$(document).ready(function() {
$('#checkboxesMain').on('click', function(e) {
if ($(this).is(':checked', true)) {
$(".checkbox").prop('checked', true);
} else {
$(".checkbox").prop('checked', false);
}
});
$('.checkbox').on('click', function() {
if ($('.checkbox:checked').length == $('.checkbox').length) {
$('#checkboxesMain').prop('checked', true);
} else {
$('#checkboxesMain').prop('checked', false);
}
});
$('.removeAll').on('click', function(e) {
var studentIdArr = [];
$(".checkbox:checked").each(function() {
studentIdArr.push($(this).attr('data-id'));
});
if (studentIdArr.length <= 0) {
alert("Choose min one item to remove.");
} else {
if (confirm("Are you sure?")) {
var stuId = studentIdArr.join(",");
$.ajax({
url: "{{url('delete-all')}}",
type: 'DELETE',
headers: {
'X-CSRF-TOKEN': $('meta[name="csrf-token"]').attr('content')
},
data: 'ids=' + stuId,
success: function(data) {
if (data['status'] == true) {
$(".checkbox:checked").each(function() {
$(this).parents("tr").remove();
});
alert(data['message']);
} else {
alert('Error occured.');
}
},
error: function(data) {
alert(data.responseText);
}
});
}
}
});
});
</script>
</html>
Now run the project.
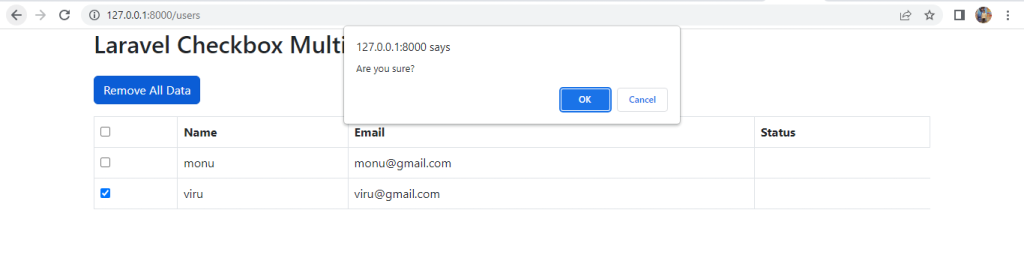
Now working successfully.