Creating a custom delete command in Laravel involves defining a new Artisan command class, specifying its signature, description, and implementing the logic to delete records based on your requirements. Here’s a step-by-step guide with explanations:
Benefits of Using this Delete Command
Creating a custom delete command in Laravel can offer several benefits, depending on the specific requirements and use cases of your application. Here are some potential benefits:
- Automation of Cleanup Tasks:
- The delete command allows you to automate cleanup tasks by regularly removing records from your database that meet certain criteria.
- This is particularly useful for removing outdated or unnecessary data, such as log entries, temporary records, or expired user sessions.
- Scheduled Data Maintenance:
- By integrating the delete command into Laravel’s task scheduler, you can schedule it to run at specific intervals (e.g., daily, weekly, or monthly).
- Scheduled data maintenance ensures that your database stays organized and optimized over time.
- Improved Performance:
- Regularly deleting unnecessary records can help maintain optimal database performance by preventing the accumulation of large amounts of data.
- Smaller, well-maintained databases generally perform better in terms of query speed and resource utilization.
- Resource Efficiency:
- Cleaning up unnecessary data contributes to resource efficiency by reducing the storage space required for your database.
- Smaller databases are easier to manage and may result in lower hosting costs.
- Data Privacy and Compliance:
- For applications handling sensitive or personal data, the delete command can assist in complying with data privacy regulations.
- Removing outdated or unnecessary user data helps maintain compliance with data protection laws.
- Database Consistency:
- The delete command helps maintain database consistency by removing records that are no longer relevant or valid.
- Consistent databases contribute to the overall reliability and integrity of your application.
Create command
php artisan make:command ClearDataCommand
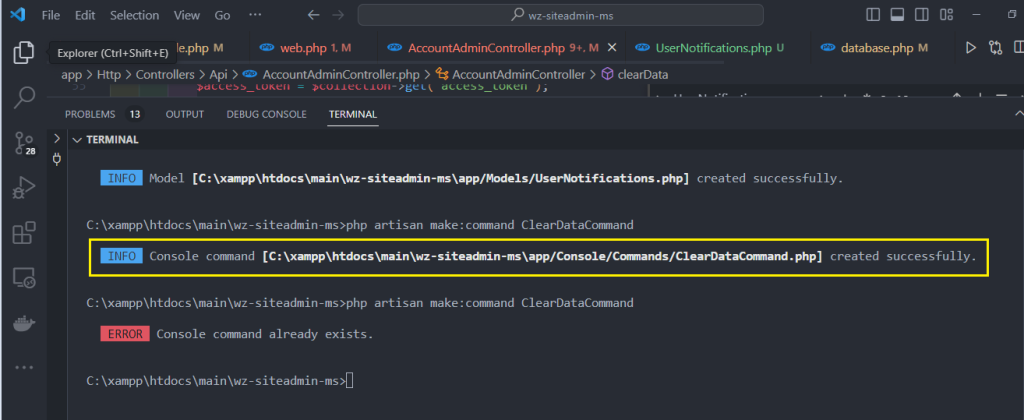
Next to go to controller and put below code
public function clearData()
{
Log::info('all delete section me hai');
$data = [];
$delteall_user = UserCount::truncate();
$AddProfileGetting = AddProfileCount::truncate();
$deleteOrgs = Userorganisation::truncate();
$deleteOrganisations = Organisation::truncate();
$delte_projects = Projects::truncate();
$AddurlCount = AddurlCount::truncate();
$getting_keyword = Keyword_count::truncate();
$TeamRating_count = TeamRating::truncate();
$WebRankCount = WebRankCount::truncate();
$pageranking = pageranking::truncate();
$socialrank = socialrank::truncate();
$PhonenumberCount = PhonenumberCount::truncate();
$Mywebaccesstoken = Mywebaccesstoken::truncate();
$Mywebaccess = Mywebaccess::truncate();
$EmailCount = EmailCount::truncate();
// $SeoPanel =SeoPanel::truncate();
$TasksBoard = TasksBoard::truncate();
$Interval = Interval::truncate();
$response = [
'success' => true,
'data' => $data,
'message' => 'Data retrieved successfully.',
];
return response()->json($response, 200);
}
Next go to ClearDataCommand.php and put below code
<?php
namespace App\Console\Commands;
use Illuminate\Console\Command;
use Illuminate\Support\Facades\Log;
use App\Http\Controllers\Api\AccountAdminController;
class ClearDataCommand extends Command
{
/**
* The name and signature of the console command.
*
* @var string
*/
protected $signature = 'clear:data';
/**
* The console command description.
*
* @var string
*/
protected $description = 'Clears data using the clearData function';
/**
* Execute the console command.
*
* @return int
*/
public function handle()
{
Log::info("clear data me in kiye hai");
$accountAdminController = new AccountAdminController();
$response = $accountAdminController->clearData();
$this->info('Account data cleared successfully.');
}
}
Next go to console -> ClearDataCommand.php
<?php
namespace App\Console;
use Illuminate\Console\Scheduling\Schedule;
use Illuminate\Foundation\Console\Kernel as ConsoleKernel;
class Kernel extends ConsoleKernel
{
/**
* Define the application's command schedule.
*
* @param \Illuminate\Console\Scheduling\Schedule $schedule
* @return void
*/
protected function schedule(Schedule $schedule)
{
// $schedule->command('inspire')->hourly();
}
/**
* Register the commands for the application.
*
* @return void
*/
protected function commands()
{
$this->load(__DIR__.'/Commands');
require base_path('routes/console.php');
}
}
Next to run below command
php artisan clear:data
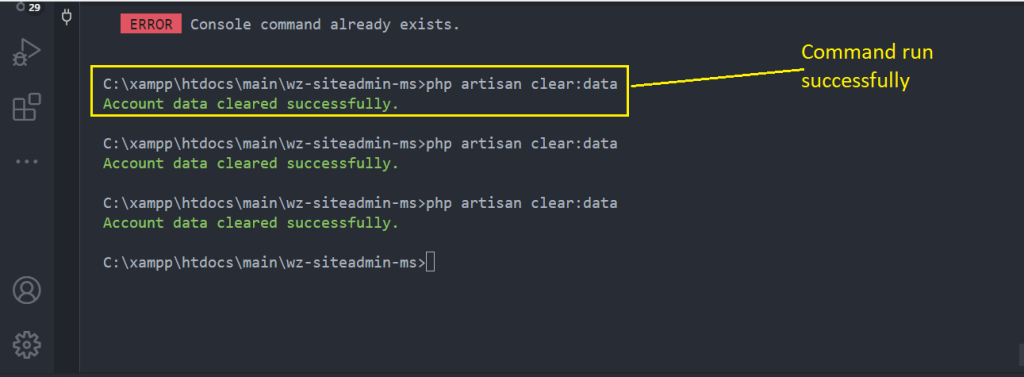
Lets check to database.
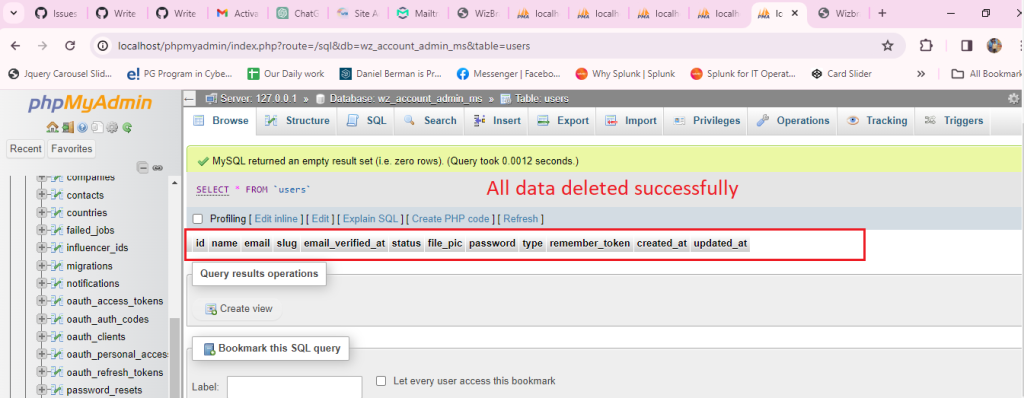
I hope its helpfull for you šš.