1st step install below package.
composer require symfony/dom-crawler
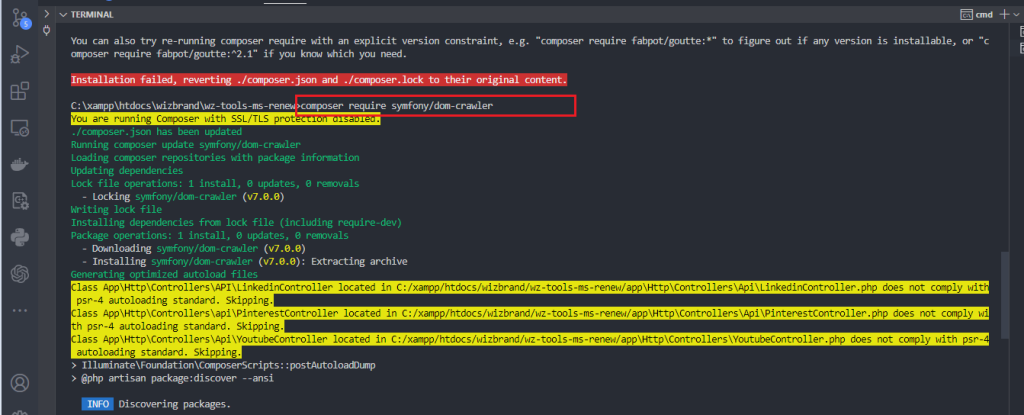
Next to create Controller
php artisan make:controller MetaTitleController
First go to route and put below code
Route::get('fetch-meta-title', [App\Http\Controllers\MetaTitleController::class, 'index']);
Next go to controller and put below code
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use Symfony\Component\DomCrawler\Crawler;
class MetaTitleController extends Controller
{
public function index()
{
return view('meta_title');
}
public function fetchHTMLMetaTitle(Request $request)
{
// Validate the URL
$request->validate([
'url' => 'required|url',
]);
// Get the URL from the request
$url = $request->input('url');
// Fetch the HTML content of the URL
$html = file_get_contents($url);
// Create a new Symfony DomCrawler
$crawler = new Crawler($html);
// Extract the main title
$mainTitleNode = $crawler->filter('title')->first();
$mainTitle = $mainTitleNode ? $mainTitleNode->text() : 'Not found';
// Extract the meta description
$metaDescriptionNode = $crawler->filter('meta[name="description"]')->first();
$metaDescription = $metaDescriptionNode ? $metaDescriptionNode->attr('content') : 'Not found';
// Extract the meta keywords
$metaKeywordsNode = $crawler->filter('meta[name="keywords"]');
$metaKeywords = $metaKeywordsNode->count() > 0 ? $metaKeywordsNode->first()->attr('content') : null;
// Return the results
return response()->json([
'mainTitle' => $mainTitle,
'metaDescription' => $metaDescription,
'metaKeywords' => $metaKeywords,
], 200);
}
}
Next go to blade page and put below code
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<style>
#main {
text-align: center;
/* background: url('amit/header.png') repeat; */
background-image: url('amit/header.png');
min-height: 535px;
transition: max-height 1.5s ease-out, min-height 1.5s ease-out;
max-height: inherit;
}
#workplace {
position: relative;
min-height: 470px;
max-width: 1000px;
margin: 0 auto;
color: #fff;
display: block;
}
#main p {
font-size: 20px;
font-weight: 200;
color: #fff;
}
#poweredByTitle {
font-weight: 200 !important;
font-size: 14px !important;
}
#UploadFile {
margin-top: 50px;
margin-bottom: 30px;
}
.filedrop {
display: flex;
flex-direction: column;
justify-content: center;
align-items: center;
z-index: 5;
position: relative;
width: 65%;
min-height: 113px;
border: 3px dashed #6dcff6;
padding: 30px 100px 30px;
margin: 0 auto;
border-radius: 10px;
box-shadow: 0 0 50px -20px #333;
box-sizing: border-box;
background: #fff;
color: #04241c;
}
.filedrop label {
font-size: 22px;
font-weight: 200;
box-sizing: content-box;
color: #04241c;
}
.filedrop label.filedrop-hint {
color: #394743;
font-size: 12px;
text-decoration: none !important;
}
.filedrop label {
font-size: 22px;
font-weight: 200;
box-sizing: content-box;
color: #04241c;
}
.mt-1 {
margin-top: 0.25rem !important;
}
#workplace h1,
#workplace .h1 {
font-size: 45px;
font-weight: 600;
text-align: center;
}
.filename {
background: #e0f4d3;
color: #7f8b77;
padding: 3px 15px;
border-radius: 6px;
margin-top: 0px;
display: inline-block;
min-width: 300px;
}
.filedrop>.fileupload>.fileRemoveLink {
color: rgba(24, 84, 128, .6);
}
.text-justify {
font-family: cursive;
}
</style>
@extends('layouts.index')
@section('content')
<div id="main">
<br>
<div id="workplace">
<h3 id="ProductTitle" class="mt-4">Fetch Meta Title Meta Descriptions and Meta Keyword</h3>
<p id="poweredByTitle" class="promo">Powered by
<a data-label="PoweredBy com" target="_blank" rel="noopener nofollow" href="https://www.wizbrand.com"
style="color: whitesmoke;">wizbrand.com</a>
</p>
<div id="WorkPlaceHolder">
<div id="UploadFile">
<div class="filedrop" id="filedrop-1">
<form id="upload-form">
@csrf
<input type="text" name="url" id="url" class="form-control" placeholder="Put your Home page url">
<input class="mt-3 btn btn-primary" type="button" id="convert-btn" value="Convert">
</form>
<div id="result">
<h4 id="mainTitle" style="color: blue"></h4>
<h5 id="metaDescription" style="color: #0eaa42"></h5>
<h6 id="metaKeywords" style="color: red"></h6>
</div>
</div>
</div>
</div>
</div>
<br>
</div>
<script type="text/javascript">
$(document).ready(function() {
$('#convert-btn').click(function() {
var form_data = new FormData($('#upload-form')[0]);
form_data.append('_token', '{{ csrf_token() }}');
$.ajax({
url: '{{ route('fetchHTMLMetaTitle') }}',
type: 'POST',
data: form_data,
processData: false,
contentType: false,
success: function(response) {
// Print the response data in the console
console.log('Main Title:', response.mainTitle);
console.log('Meta Description:', response.metaDescription);
console.log('Meta Keywords:', response.metaKeywords);
$('#mainTitle').text("Meta Title : " + response.mainTitle);
$('#metaDescription').text("Meta Description Title : " + response.metaDescription);
$('#metaKeywords').text("Meta Keyword : " + response.metaKeywords);
},
error: function(xhr, textStatus, errorThrown) {
alert('Error: ' + errorThrown);
}
});
});
});
</script>
@endsection
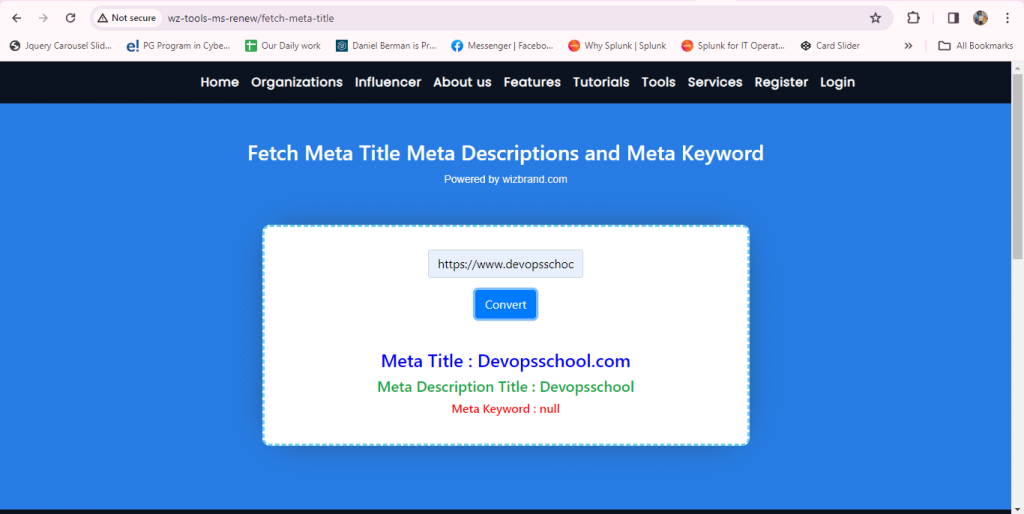
Now successfully showing meta title and meta descriptions.