In this tutorial we’re going to learn how to create functionality active and inactive status in laravel. We can implement change status on click on button using ajax with bootstrap toggle button. Here we will update user status active inactive with boolean data type with 0 and 1.
First install Laravel project
composer create-project laravel/laravel example-app
Next to Generate Authentication
composer requires laravel/ui
php artisan ui bootstrap
php artisan ui bootstrap --auth
Next to run below code
npm install
npm run dev
Next go to migration file and put 1 column
$table->boolean('status')->default(0);
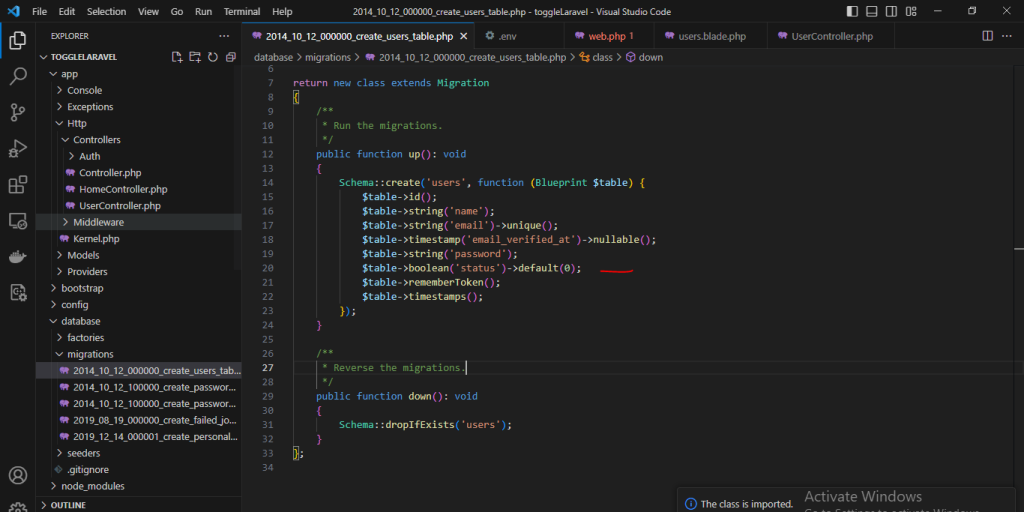
Now migrate the table
php artisan migrate
Next to create Controller file using below code.
php artisan make:controller UserController

Next got to controller and put below code.
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\User;
class UserController extends Controller
{
/**
* Responds with a welcome message with instructions
*
* @return \Illuminate\Http\Response
*/
public function index()
{
$users = User::get();
return view('users',compact('users'));
}
/**
* Responds with a welcome message with instructions
*
* @return \Illuminate\Http\Response
*/
public function changeStatus(Request $request)
{
$user = User::find($request->user_id);
$user->status = $request->status;
$user->save();
return response()->json(['success'=>'Status change successfully.']);
}
}
Next to create view page
resources/views/users.blade.php
Next go to users.blade.php and put below code.
<!DOCTYPE html>
<html>
<head>
<title>Laravel Update User Status Using Toggle Button Example</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.4.1/jquery.min.js" ></script>
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/twitter-bootstrap/3.3.7/css/bootstrap.min.css" />
<link href="https://gitcdn.github.io/bootstrap-toggle/2.2.2/css/bootstrap-toggle.min.css" rel="stylesheet">
<script src="https://gitcdn.github.io/bootstrap-toggle/2.2.2/js/bootstrap-toggle.min.js"></script>
</head>
<body>
<br><br>
<div class="container mt-5">
<h1>Laravel Update User Status Using Toggle Button Example </h1>
<table class="table table-bordered">
<thead>
<tr>
<th>Name</th>
<th>Email</th>
<th>Status</th>
</tr>
</thead>
<tbody>
@foreach($users as $user)
<tr>
<td>{{ $user->name }}</td>
<td>{{ $user->email }}</td>
<td>
<input data-id="{{$user->id}}" class="toggle-class" type="checkbox" data-onstyle="success" data-offstyle="danger" data-toggle="toggle" data-on="Active" data-off="InActive" {{ $user->status ? 'checked' : '' }}>
</td>
</tr>
@endforeach
</tbody>
</table>
</div>
</body>
<script>
$(function() {
$('.toggle-class').change(function() {
var status = $(this).prop('checked') == true ? 1 : 0;
var user_id = $(this).data('id');
$.ajax({
type: "GET",
dataType: "json",
url: '/changeStatus',
data: {'status': status, 'user_id': user_id},
success: function(data){
console.log(data.success)
}
});
})
})
</script>
</html>
Next go to web.php and put below code.
Route::get('users', [App\Http\Controllers\UserController::class, 'index']);
Route::get('changeStatus', [App\Http\Controllers\UserController::class, 'changeStatus']);
Now run the project using below code
php artisan serve
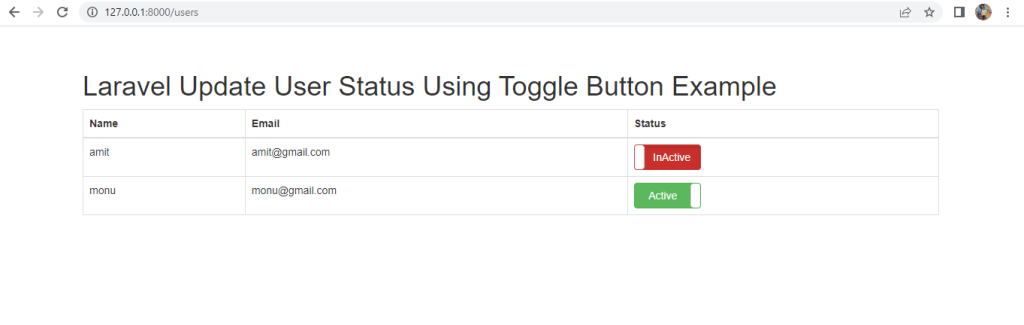
Let’s got to database and check status would be update or not.
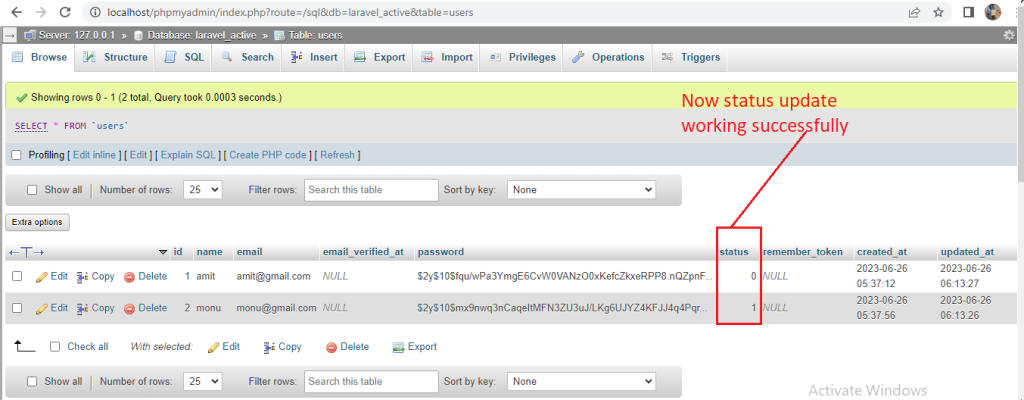