What is Flask ?
Flask is a lightweight and flexible web framework written in Python. It is designed to make getting started with web development quick and easy, with the ability to scale up to complex applications. Flask provides tools and libraries to help you build web applications and APIs in Python, with a focus on simplicity and minimalism.
Key features of Flask include:
- Minimalistic: Flask is designed to be lightweight and unopinionated, allowing developers to choose the tools and libraries they want to use.
- Routing: Flask provides a simple and intuitive routing system, allowing developers to define URL routes and map them to Python functions (view functions) that handle HTTP requests.
- Templates: Flask includes a template engine called Jinja2, which allows developers to write HTML templates with dynamic content using Python-like syntax.
- HTTP Request Handling: Flask provides built-in support for handling HTTP requests, including parsing request data, handling form submissions, and managing sessions.
- Extensions: Flask has a rich ecosystem of extensions that add additional functionality to the framework, such as database integration, authentication, and security features.
- Development Server: Flask includes a built-in development server that makes it easy to run and test your web applications locally during development.
- RESTful APIs: Flask is well-suited for building RESTful APIs, allowing developers to create web services that communicate with other applications or clients over HTTP.
Run below command
python -m venv venv
.\venv\Scripts\activate
python -m pip install --upgrade pip
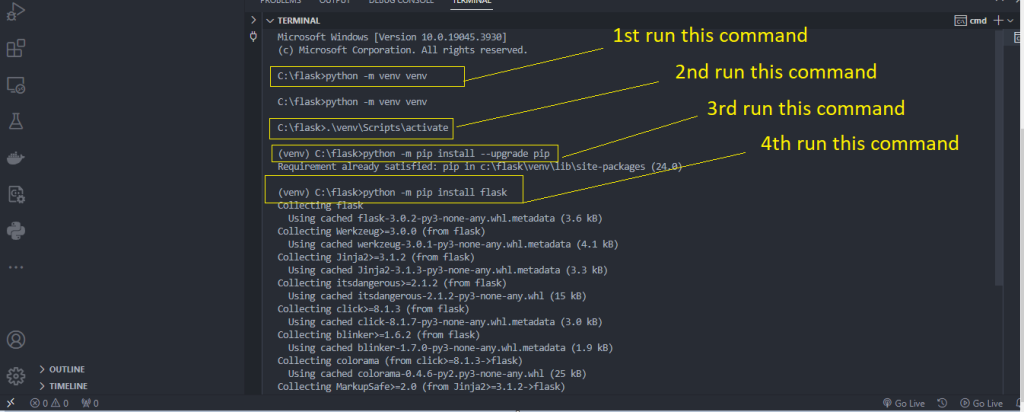
Next to install Flask
pip install flask
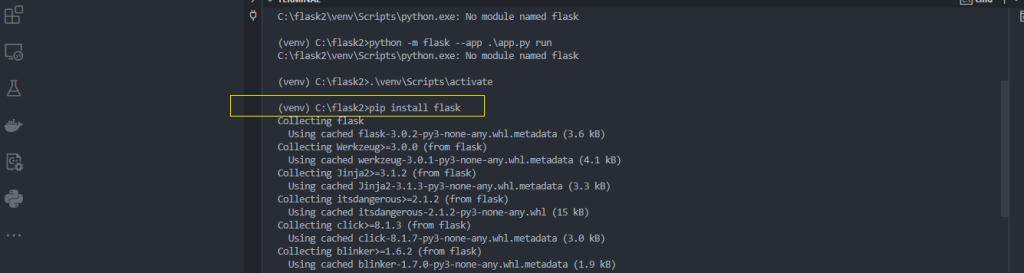
Next to create new file app.py and put below code
touch app.py
from flask import Flask
app = Flask(__name__)
@app.route("/")
def home():
return "Hellow world"
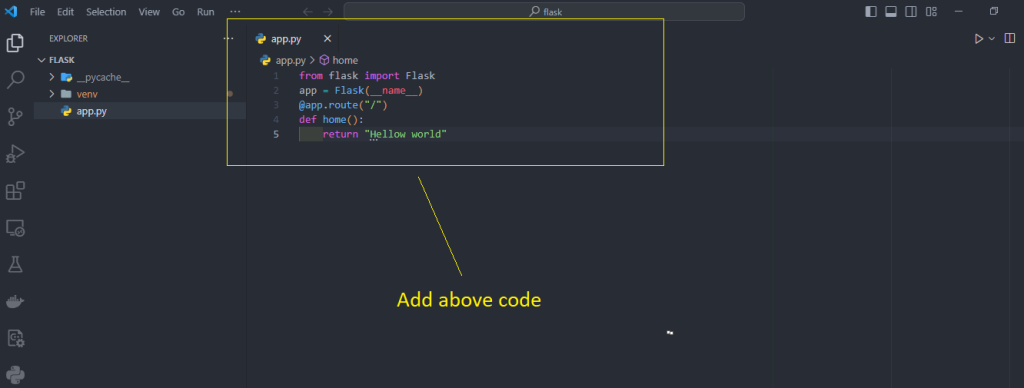
Next to run below command for serve
python -m flask --app .\app.py run
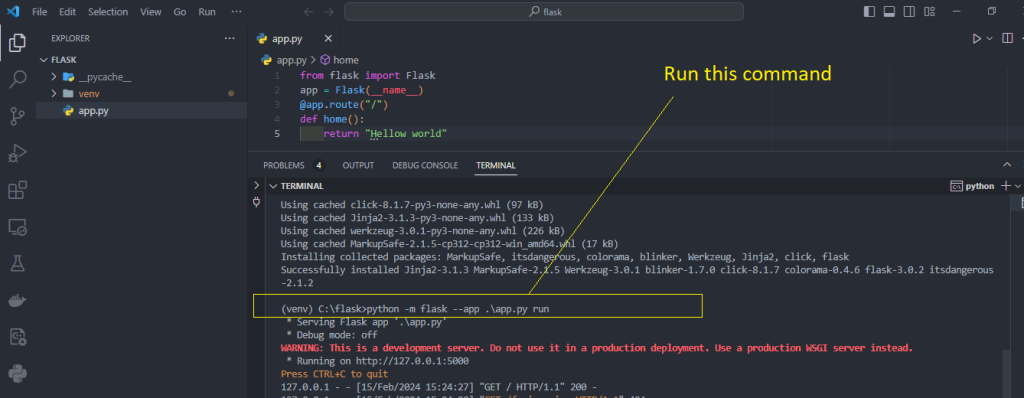
Next to open development server and check
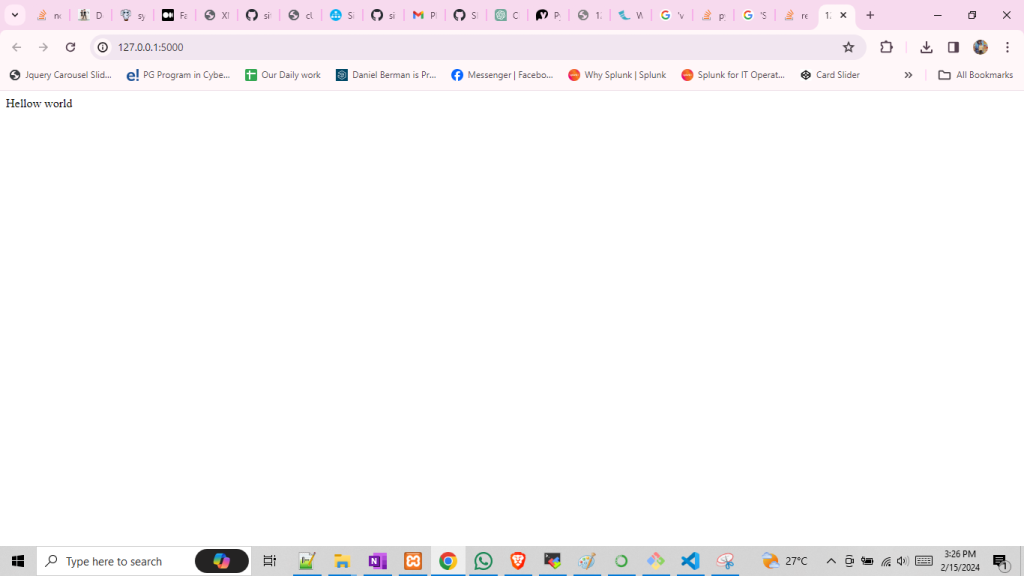