What is Module in Node Js?
In Node.js, a module is essentially a reusable piece of code that encapsulates related functionality. Modules help in organizing code into separate files or packages, making it easier to manage, reuse, and maintain. There are different types of modules in Node.js:
- Creating a Module: To create a module, you simply define your functionality in a separate file. This file can contain variables, functions, classes, or any other JavaScript code. By default, everything defined in a file in Node.js is scoped to that file, meaning it’s not automatically accessible from other files unless explicitly exported.
- Exporting from a Module: To make functionality available outside the module, you use the
module.exports
orexports
object to export the desired variables, functions, or objects. This makes them accessible to other modules thatrequire
or import them. - Importing/Requiring a Module: To use functionality from a module in another file, you use the
require()
function to import the module. This function returns the exported functionality from the module, allowing you to use it within your code.
Example:-
1st create math.js file and put below code
function add(a,b){
return a + b;
}
function sub(a,b){
return a-b;
}
module.exports = {
add,sub
}
Next to create hellow.js file and put below code
const math = require("./math");
console.log("math value is ",math);
Next to run below command
npm run start
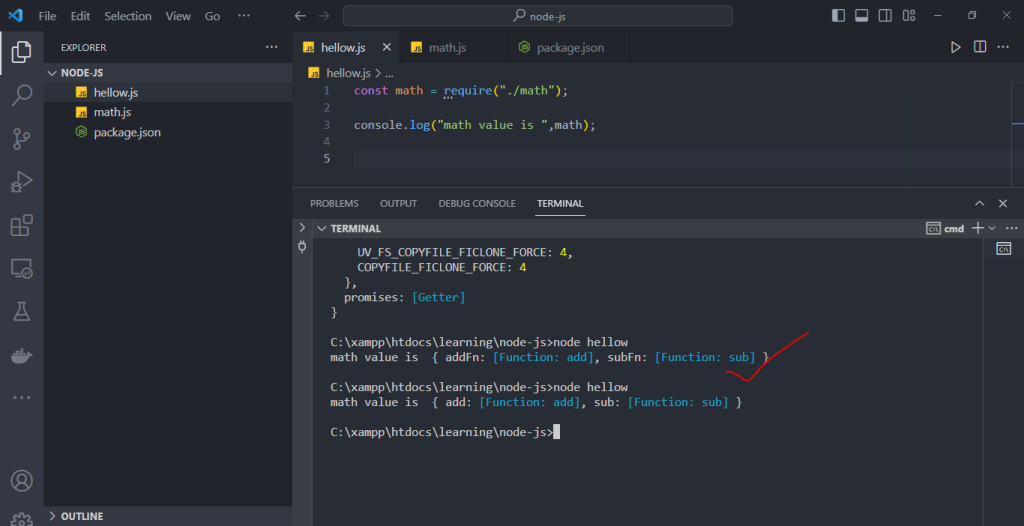