In this tutorial we’re going to learn how to use inharitance in php with example.
Inheritance is a way to create a new class that is a modified version of an existing class. The new class inherits properties and methods from the existing class and can also have its own additional properties and methods.
Example :-
<?php
class employee {
public $name;
public $age;
public $salary;
function __construct($n, $a, $s,){
$this->name = $n;
$this->age = $a;
$this->salary = $s;
}
function info(){
echo"<h3>employee Profile</h3>";
echo "employee name :" .$this->name."<br>";
echo "employee age :" .$this->age."<br>";
echo "employee salary :" .$this->salary."<br>";
}
}
$e1 = new employee("Ram",25,2000);
$e1->info();
?>
Output :-
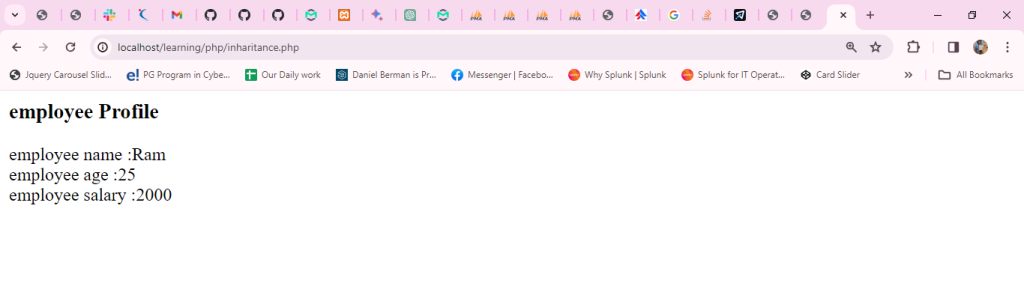