In this tutorial we’re going to share how to use slice function in laravel with example.
In PHP, the array_slice()
function is used to extract a slice of an array. It returns a portion of the array based on the given start and length parameters. The syntax of the array_slice()
function is as follows:
$array
: The input array to be sorted. It is passed by reference and will be modified in place.$flags
: Optional. Specifies the sorting behavior. It can take one of the following constants:SORT_REGULAR
: Compare items normally (default).SORT_NUMERIC
: Compare items numerically.SORT_STRING
: Compare items as strings.
Example:-
<?php
$array = ['apple', 'banana', 'cherry', 'date', 'elderberry'];
$slice = array_slice($array, 0,2);
print_r($slice);
?>
Ouput:-
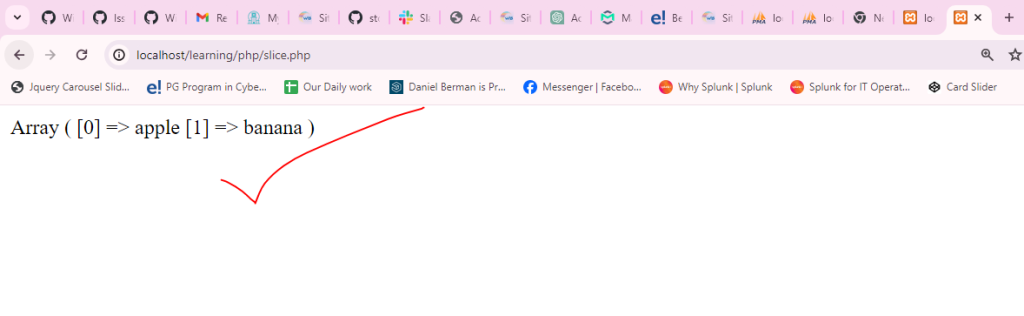
rsort function
The rsort()
function in PHP is used to sort an array in reverse order. It sorts the elements of the array in descending order, with the highest values coming first. The syntax of the rsort()
function is:
Example:-
<?php
$all_data = [23,45,455,645,78,798];
rsort($all_data);
print_r($all_data);
?>,
Output:-
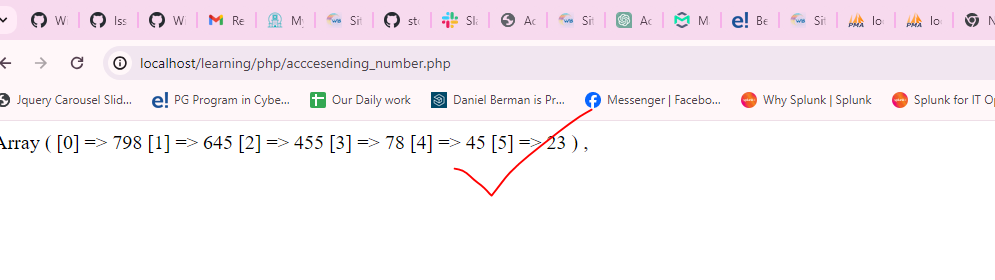