In this tutorial we are going to learn how to setup sweet alert when crud will be performing.
Create New Controller
php artisan make:controller StudentController
We have to create specific functions that will add sweet alert; therefore, enter into the app/Http/Controllers/StudentController.php file and make sure to replace the current code with the following code.
<?php
namespace App\Http\Controllers;
use App\Models\User;
use DB;
use Illuminate\Http\Request;
use Illuminate\Support\Facades\Hash;
class StudentController extends Controller
{
public function usersList()
{
$students = User::all();
return view('home', compact('students'));
}
public function removeUser($id)
{
$delete = User::where('id', $id)->delete();
if ($delete == $id) {
$success = true;
$message = "Student successfully removed!";
} else {
$success = true;
$message = "Student does not exist!";
}
// Return response
return response()->json([
'success' => $success,
'message' => $message,
]);
}
}
Build View Template
Enter into the resources/views/ directory; here you have to create a new file and put below cdn.
<script src="//cdn.jsdelivr.net/npm/sweetalert2@11"></script>
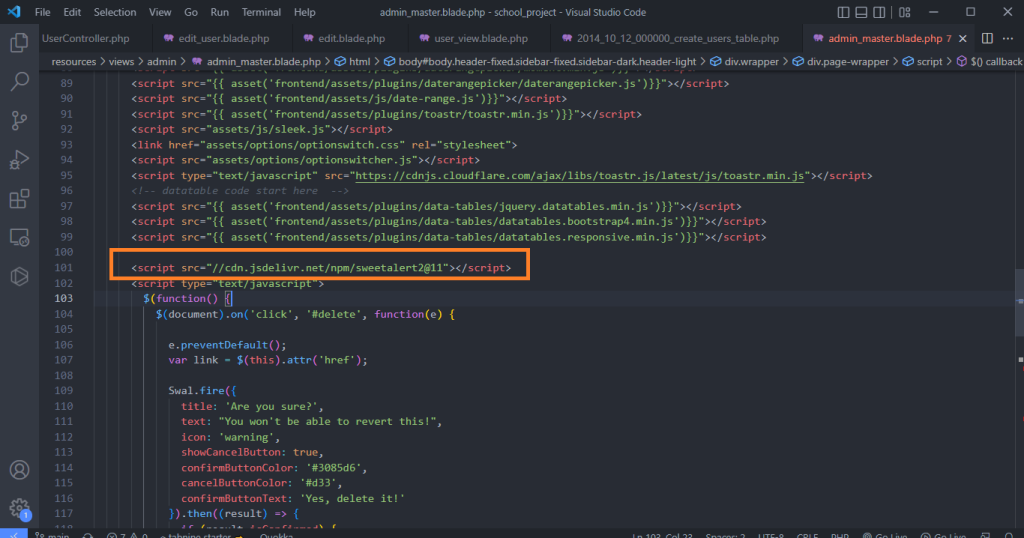
Next put below script in your code.
<script type="text/javascript">
$(function() {
$(document).on('click', '#delete', function(e) {
e.preventDefault();
var link = $(this).attr('href');
################################
Swal.fire({
title: 'Are you sure?',
text: "You won't be able to revert this!",
icon: 'warning',
showCancelButton: true,
confirmButtonColor: '#3085d6',
cancelButtonColor: '#d33',
confirmButtonText: 'Yes, delete it!'
}).then((result) => {
if (result.isConfirmed) {
window.location.href = link
Swal.fire(
'Deleted!',
'Your file has been deleted.',
'success'
)
}
})
######################
});
});
</script>
Next go to your blade page where you want to show alert and paste below id
<a class="btn btn-warning" href="{{ route('users.delete',$users->id)}}" id="delete">Delte</a>
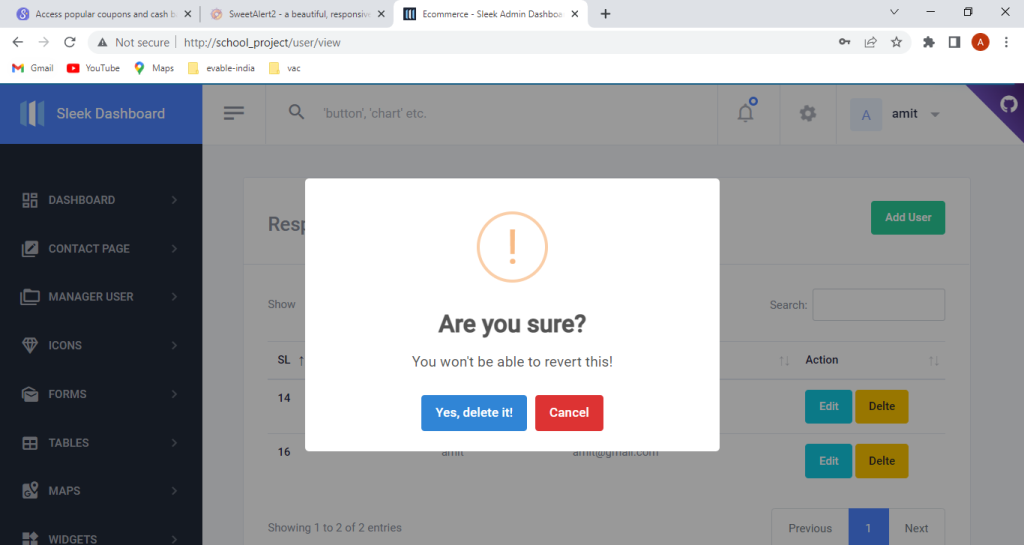
Now sweet alert coming successfully thanks happy coding.
[…] How to Show Notification using Sweet Alert in Laravel 9 […]