In this tutorial we’re going to learn how to send notification if someone register on our software then send him notification.
Install laravel project
composer create-project laravel/laravel:^9.0 notification_email
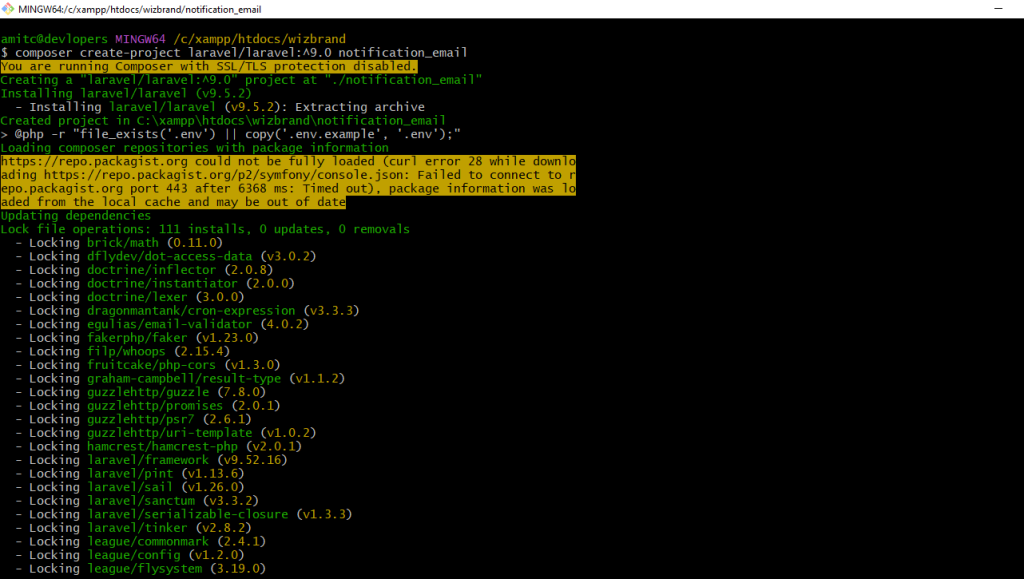
Next to create migration file using below command
php artisan notification:table
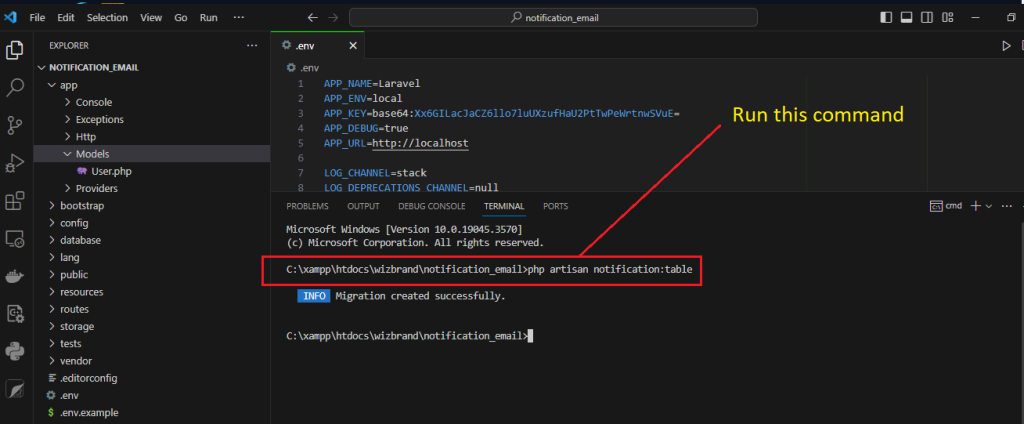
Next migrate the table
php artisan migrate
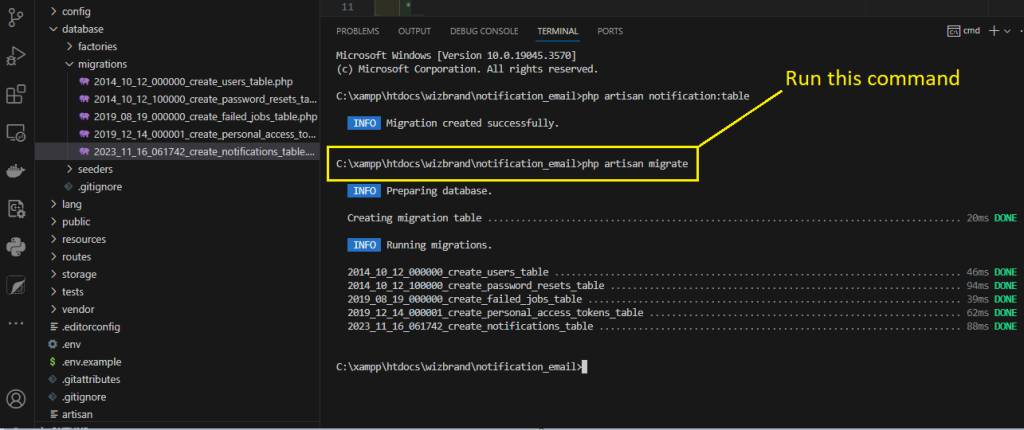
Next to generate authentication
composer require laravel/ui
php artisan ui bootstrap
php artisan ui bootstrap --auth
Next to run below command
npm install && npm run dev
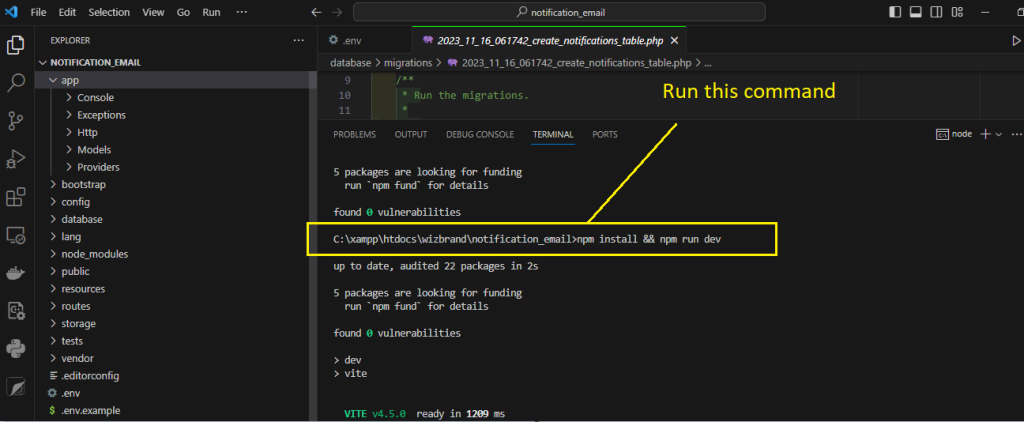
Next to start the server
php artisan serve
And do register and login
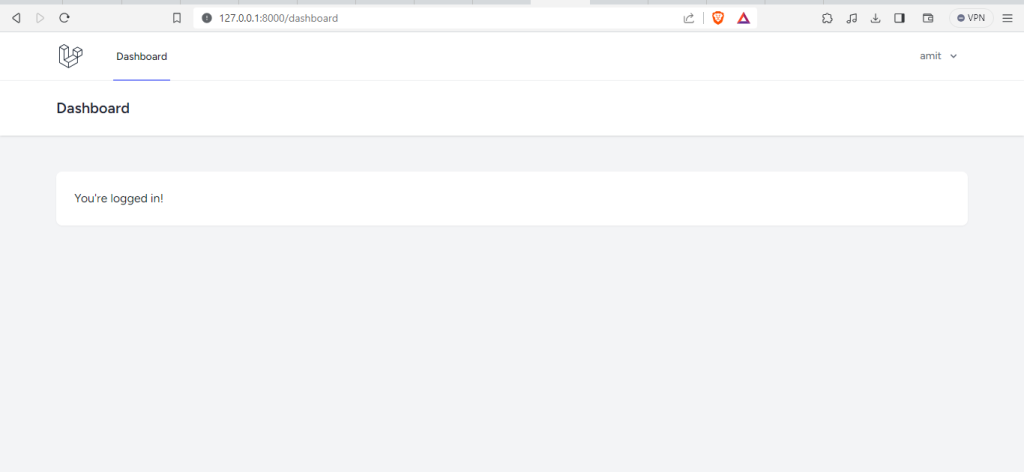
Next to run below command
php artisan make:notification UserFollowNotification
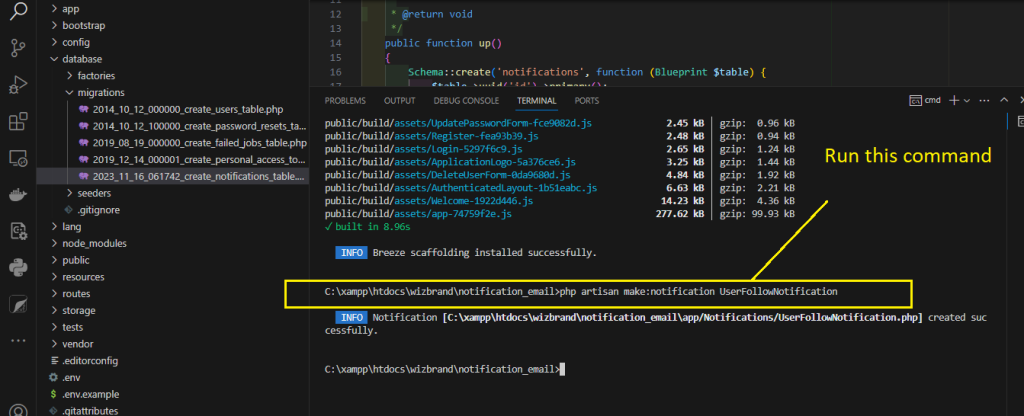
Next go to UserFollowNotification and put below code
<?php
namespace App\Notifications;
use Illuminate\Bus\Queueable;
use Illuminate\Contracts\Queue\ShouldQueue;
use Illuminate\Notifications\Messages\MailMessage;
use Illuminate\Notifications\Notification;
class UserFollowNotification extends Notification
{
use Queueable;
public $user;
/**
* Create a new notification instance.
*/
public function __construct($user)
{
$this->user = $user;
}
/**
* Get the notification's delivery channels.
*
* @return array<int, string>
*/
public function via(object $notifiable): array
{
return ['database'];
}
/**
* Get the mail representation of the notification.
*/
public function toMail(object $notifiable): MailMessage
{
return (new MailMessage)
->line('The introduction to the notification.')
->action('Notification Action', url('/'))
->line('Thank you for using our application!');
}
/**
* Get the array representation of the notification.
*
* @return array<string, mixed>
*/
public function toArray(object $notifiable): array
{
return [
'user_id'=> $this->user['id'],
'name'=> $this->user['name'],
'email'=> $this->user['email']
];
}
}
Next go to profilecontroller and put below code
public function notify(){
if (auth()->user()) {
$user = auth()->user();
Log::info("user me kya aa rha hai: " . $user);
auth()->user()->notify(new UserFollowNotification($user));
}
dd('done');
}
Next go to web.php and put below code
Route::get('/notify', [ProfileController::class, 'notify']);
Next to hit this route
Route::get('/notify', [ProfileController::class, 'notify']);
Next go to blade page where you want to show the notification I have put the dashboard.blade.php
<x-app-layout>
<x-slot name="header">
<h2 class="font-semibold text-xl text-gray-800 leading-tight">
{{ __('Dashboard') }}
</h2>
</x-slot>
<div class="py-12">
<div class="max-w-7xl mx-auto sm:px-6 lg:px-8">
<div class="bg-white overflow-hidden shadow-sm sm:rounded-lg">
<div class="p-6 text-gray-900 bg-white border-b" style="color: blue;">
@foreach (auth()->user()->unreadnotifications as $notification)
<div class="bg-blue-300 p-3 m-2">
<b> {{ $notification->data['name']}}</b> started following you !!!
<a href="{{ route('marksread',$notification->id)}}" class="p-2 bg-red-400 rounded-lg" style="color: red;">mark as read</a>
</div>
@endforeach
</div>
</div>
</div>
</div>
</x-app-layout>
Next go to web.php and put below code for mark as unread message
Route::get('/marksread/{id}', [ProfileController::class, 'markasread'])->name('marksread');
public function markasread($id){
if($id){
auth()->user()->unreadNotifications->where('id',$id)->markAsRead();
}
return back();
}
Next run the project and login
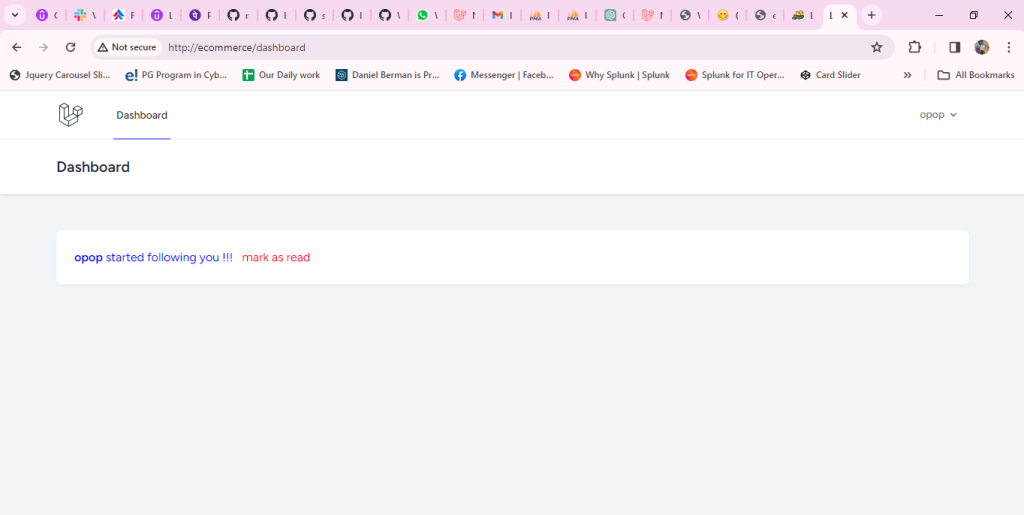
Now notification coming successfully.
[…] How to send Notifications With Database In Laravel ? […]