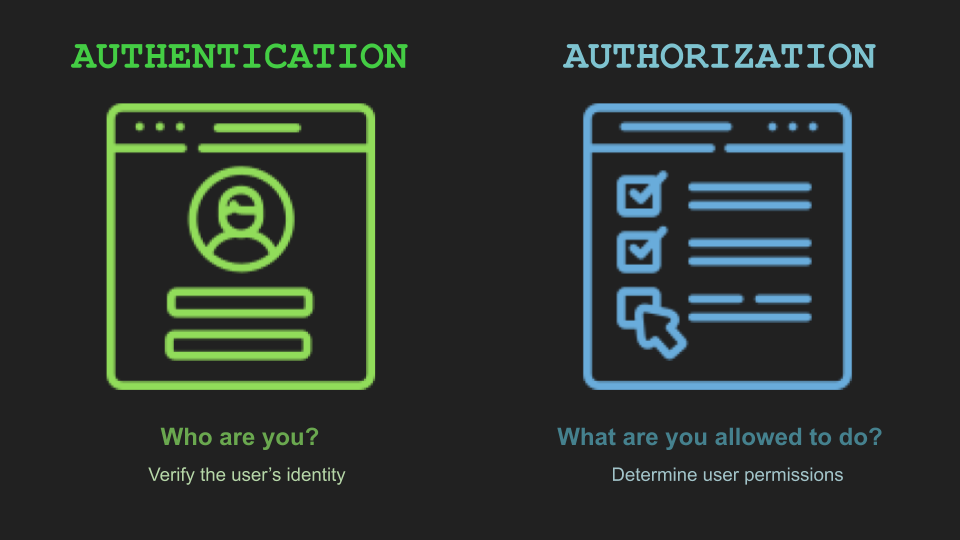
- Authentication:
- Definition: Authentication is the process of verifying the identity of a user, system, or process. It ensures that a user is who they claim to be.
- Example in Laravel:In Laravel, authentication is the process of validating the credentials of a user, typically their email and password, against the stored credentials in a database. Laravel provides built-in authentication mechanisms that make it easy to get a fully functional authentication system up and running quickly.
- Code Example:To authenticate a user, you can use Laravel’s
Auth
facade. Here’s an example of how to authenticate a user:php
if (Auth::attempt(['email' => $email, 'password' => $password])) { // The user is authenticated return redirect('/dashboard'); }
- Authorization:
- Definition: Authorization is the process of granting or denying access to specific resources or actions based on the authenticated user’s permissions and roles.
- Example in Laravel:In Laravel, authorization is typically done through middleware, policies, or gates. These mechanisms allow you to define who is allowed to perform certain actions or access specific routes or resources in your application.
- Code Example:Using middleware, you can restrict access to certain routes based on the user’s role. For example, a user with the role “Admin” might have access to an admin dashboard, while a regular user might not.php
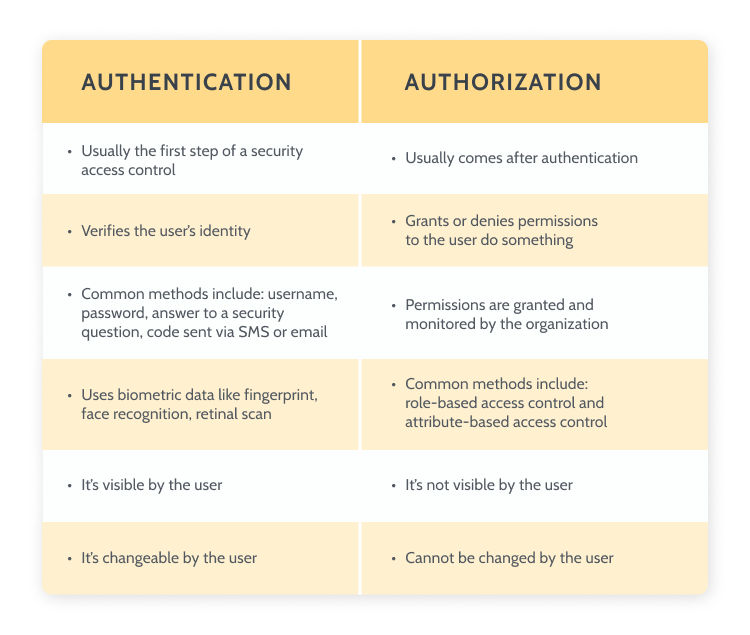
Route::middleware(['auth', 'admin'])->group(function () { Route::get('/admin/dashboard', 'AdminController@dashboard'); });
Summary:
- Authentication is about verifying the identity of a user.
- Authorization is about granting or denying access to specific resources or actions based on the authenticated user’s permissions.
[…] What are the Difference Between Authentication and Authorization in Laravel ? […]